form.validateFields().then((value)参数类型ts
时间: 2023-06-13 19:04:32 浏览: 115
`form.validateFields()` 方法返回一个 `Promise` 对象,其 `then` 方法接收一个回调函数,该回调函数的参数类型是一个对象,该对象的属性和值对应表单中的字段名和对应的值。在 TypeScript 中,可以使用以下方式定义该回调函数的参数类型:
```typescript
interface FormValues {
[key: string]: any;
}
form.validateFields().then((values: FormValues) => {
// 处理表单数据
});
```
其中,`FormValues` 是一个接口,它表示一个对象,该对象的属性名是字符串类型,属性值可以是任意类型。这样定义可以使得回调函数的参数类型更加灵活,可以适应不同表单的字段名和字段值的类型。
相关问题
form.validateFields()
`form.validateFields()`是Ant Design表单组件中的一个方法,用于验证表单中的所有字段。它会遍历表单中的所有字段,依次调用每个字段的校验规则进行验证,并返回一个Promise对象,验证结果会包含所有字段的验证结果。
`form.validateFields()`方法的语法如下:
```javascript
form.validateFields([fieldNames: string[]], [options: object], callback(errors, values))
```
其中,`fieldNames`参数是一个可选的字符串数组,指定需要验证的字段名称。如果未指定该参数,则会验证表单中的所有字段。
`options`参数是一个可选的对象,用于指定验证选项。常用的选项包括:
- `force`: 是否强制验证,即使字段未被修改也会进行验证,默认为`false`。
- `scroll`: 是否在验证失败时滚动到第一个验证失败的字段,默认为`true`。
- `firstFields`: 在验证失败时,优先滚动到指定的字段,默认为`undefined`。
`callback`参数是一个回调函数,用于处理验证结果。它接受两个参数:`errors`和`values`。`errors`是一个对象,包含了所有验证失败的字段以及对应的错误信息;`values`是一个对象,包含了所有字段的最新值。
下面是一个简单的示例,演示如何使用`form.validateFields()`方法验证表单:
```javascript
import { Form, Input, Button } from 'antd';
const DemoForm = () => {
const [form] = Form.useForm();
const onFinish = (values) => {
console.log('Received values of form: ', values);
};
const onFinishFailed = (errors) => {
console.log('Failed:', errors);
};
const handleValidate = () => {
form.validateFields().then((values) => {
console.log('Success:', values);
onFinish(values);
}).catch((errors) => {
console.log('Failed:', errors);
onFinishFailed(errors);
});
};
return (
<Form
form={form}
onFinish={onFinish}
onFinishFailed={onFinishFailed}
>
<Form.Item
name="username"
label="Username"
rules={[{ required: true, message: 'Please input your username!' }]}
>
<Input />
</Form.Item>
<Form.Item
name="password"
label="Password"
rules={[{ required: true, message: 'Please input your password!' }]}
>
<Input.Password />
</Form.Item>
<Form.Item>
<Button type="primary" onClick={handleValidate}>Validate</Button>
</Form.Item>
</Form>
);
};
```
在这个示例中,我们使用了Ant Design的`Form`组件,并在组件外部定义了一个`handleValidate`函数,用于执行表单验证。当用户点击“验证”按钮时,该函数会调用`form.validateFields()`方法进行表单验证。如果验证通过,它会调用`onFinish`回调函数,将验证结果传递给该函数进行处理;如果验证失败,则会调用`onFinishFailed`回调函数。
注意,`form.validateFields()`方法返回的是一个Promise对象,您需要使用`.then()`和`.catch()`方法来处理验证结果。另外,在使用Ant Design的`Form`组件时,您需要使用`Form.useForm()`方法来获取表单实例。
form.validatefields不运行
form.validateFields()是Ant Design中的一个方法,用于验证表单字段的有效性。如果在使用过程中发现该方法不起作用,可能是由于以下几个原因之一:
1. 表单字段未正确绑定:使用form.getFieldDecorator()方法绑定表单字段时,需要传入标识字段名和规则等参数,确保正确绑定。
2. 表单组件未正确包裹:在使用getFieldDecorator()方法时,需要将表单组件正确包裹在其内部,如Input、Select等,确保getFieldDecorator()可以获取到组件的值。
3. 异步验证问题:validateFields()方法默认是异步验证的,如果在调用该方法后立即对表单字段进行操作,可能导致没有得到验证结果。可以通过在validateFields()方法的回调函数中进行后续操作来解决。
以下是一个示例代码,演示了如何正确使用form.validateFields()方法:
```javascript
import React, { Component } from 'react';
import { Form, Input, Button } from 'antd';
class MyForm extends Component {
handleSubmit = (e) => {
e.preventDefault();
this.props.form.validateFields((err, values) => {
if (!err) {
console.log('Received values of form: ', values);
}
});
}
render() {
const { getFieldDecorator } = this.props.form;
return (
<Form onSubmit={this.handleSubmit}>
<Form.Item>
{getFieldDecorator('username', {
rules: [{ required: true, message: 'Please input your username!' }],
})(
<Input placeholder="Username" />
)}
</Form.Item>
<Form.Item>
{getFieldDecorator('password', {
rules: [{ required: true, message: 'Please input your password!' }],
})(
<Input type="password" placeholder="Password" />
)}
</Form.Item>
<Form.Item>
<Button type="primary" htmlType="submit">
Submit
</Button>
</Form.Item>
</Form>
);
}
}
const WrappedMyForm = Form.create({ name: 'my_form' })(MyForm);
export default WrappedMyForm;
```
在上述示例中,我们创建了一个表单,其中包含了用户名和密码两个字段。在提交表单时,通过调用form.validateFields()方法来验证字段的有效性。如果验证通过,则打印表单的值。
希望以上解答对您有帮助。如果问题仍然存在,请提供更多的代码或信息,以便我们能够更准确地帮助您解决问题。
相关推荐
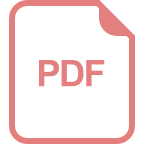












