Python代码爬取北京地铁客流量
时间: 2023-11-05 17:49:42 浏览: 82
首先,我们需要准备好以下工具:
- requests 库,用于发送 HTTP 请求和获取响应;
- BeautifulSoup 库,用于解析 HTML 页面;
- pandas 库,用于处理数据和生成表格;
- matplotlib 库,用于绘制图表。
接下来,我们可以按照以下步骤爬取北京地铁客流量:
1. 打开北京市地铁客流量查询页面(http://www.bjsubway.com/e/action/ListInfo/?classid=39),发起 GET 请求,并获取响应内容。
```python
import requests
url = "http://www.bjsubway.com/e/action/ListInfo/?classid=39"
response = requests.get(url)
html = response.content
```
2. 使用 BeautifulSoup 库解析 HTML 页面,并找到包含客流量数据的表格。
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html, "html.parser")
table = soup.find("table", class_="table01")
```
3. 遍历表格中的每一行,提取每个站点的客流量数据,并将其保存到一个字典中。
```python
data = {}
for tr in table.find_all("tr")[1:]:
td_list = tr.find_all("td")
station = td_list[1].text.strip()
in_count = int(td_list[2].text.strip())
out_count = int(td_list[3].text.strip())
total_count = in_count + out_count
data[station] = {
"in_count": in_count,
"out_count": out_count,
"total_count": total_count
}
```
4. 将数据保存到一个 CSV 文件中,并使用 pandas 库读取该文件并生成一个数据框。
```python
import csv
import pandas as pd
with open("beijing_subway.csv", "w", newline="", encoding="utf-8") as f:
writer = csv.writer(f)
writer.writerow(["station", "in_count", "out_count", "total_count"])
for station, count_dict in data.items():
writer.writerow([station, count_dict["in_count"], count_dict["out_count"], count_dict["total_count"]])
df = pd.read_csv("beijing_subway.csv")
```
5. 利用数据框生成图表,展示各个站点的客流量情况。
```python
import matplotlib.pyplot as plt
import matplotlib.font_manager as fm
# 设置中文字体
font_path = "C:\Windows\Fonts\SimHei.ttf"
font_prop = fm.FontProperties(fname=font_path, size=14)
# 绘制柱状图
plt.figure(figsize=(12, 6))
plt.bar(df.station, df.total_count)
plt.title("北京市地铁客流量情况", fontproperties=font_prop)
plt.xlabel("站点", fontproperties=font_prop)
plt.ylabel("客流量(人次)", fontproperties=font_prop)
plt.xticks(rotation=90, fontproperties=font_prop)
plt.grid(axis="y")
plt.show()
```
相关推荐
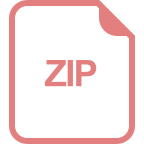
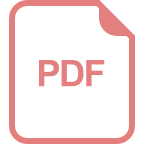














