python怎么用多线程实现对方法超时监控
时间: 2023-12-24 22:05:45 浏览: 29
可以使用Python的`threading`模块来实现对方法超时监控。具体步骤如下:
1. 定义一个装饰器函数`timeout`,该函数接受一个超时时间参数`seconds`和一个函数参数`_func`,返回一个新的函数`func`。
2. 在`func`函数中启动一个新的线程`thread`,并在该线程中执行函数`_func`。
3. 在`func`函数中调用`thread.join`方法,等待线程执行完毕,或者超时时间到达,如果超时时间到达,就终止线程的执行。
4. 如果线程执行完毕,就返回函数`_func`的返回值,如果线程超时终止,就抛出一个超时异常。
下面是一个具体的实现代码示例:
```python
import threading
import functools
import time
class TimeoutException(Exception):
pass
def timeout(seconds):
def decorator(func):
@functools.wraps(func)
def func_wrapper(*args, **kwargs):
def thread_wrapper():
func_wrapper.result = func(*args, **kwargs)
func_wrapper.result = None
thread = threading.Thread(target=thread_wrapper)
thread.start()
thread.join(seconds)
if thread.is_alive():
thread._stop()
raise TimeoutException('Function {} timed out after {} seconds'.format(func.__name__, seconds))
return func_wrapper.result
return func_wrapper
return decorator
@timeout(3)
def my_func():
time.sleep(5)
return "Hello"
try:
result = my_func()
print(result)
except TimeoutException as e:
print(e)
```
在上面的代码中,我们定义了一个`timeout`装饰器函数,它接受一个超时时间参数`seconds`和一个函数参数`_func`,并返回一个新的函数`func`。在`func`函数中,我们启动了一个新的线程`thread`,并在该线程中执行函数`_func`。然后在`func`函数中调用`thread.join`方法,等待线程执行完毕,或者超时时间到达。如果线程执行完毕,就返回函数`_func`的返回值,如果线程超时终止,就抛出一个超时异常`TimeoutException`。最后,我们定义了一个`my_func`函数,并在其上面应用了`timeout`装饰器,来实现对该函数的超时监控。
相关推荐
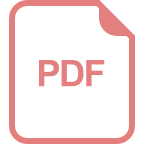
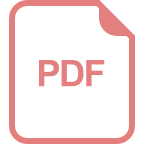
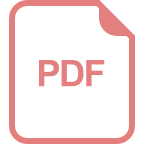














