1.创建二维数组等数据结构用来表示进程对资源的使用情况,相关数据包括进程名称、进程数目、资源数目、最大需求数目、已分配数目、仍需数目及当前状态下可利用数目等。 2.在用户输入所有初始化信息后,首先对信息以矩阵形式输出,再输入一组可用资源数目,此时系统判断是否存在安全序列,若有则输出一个安全序列;若无,则表示初始化不正确,请重新输入。用java实现
时间: 2024-03-14 13:46:11 浏览: 16
好的,我可以为您提供一个用Java实现银行家算法的示例代码,具体如下:
```
import java.util.Scanner;
public class BankerAlgorithm {
private int[][] allocation; // 进程已分配资源数
private int[][] max; // 进程最大需求数
private int[][] need; // 进程还需要资源数
private int[] available; // 系统可用资源数
private int numOfProcess; // 进程数
private int numOfResource; // 资源数
private boolean[] finish; // 进程是否完成标记
// 初始化各种数据结构
public void init() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入进程数目:");
numOfProcess = scanner.nextInt();
System.out.print("请输入资源数目:");
numOfResource = scanner.nextInt();
allocation = new int[numOfProcess][numOfResource];
max = new int[numOfProcess][numOfResource];
need = new int[numOfProcess][numOfResource];
available = new int[numOfResource];
finish = new boolean[numOfProcess];
// 输入各进程已分配资源数
System.out.println("请输入各进程已分配资源数:");
for (int i = 0; i < numOfProcess; i++) {
for (int j = 0; j < numOfResource; j++) {
allocation[i][j] = scanner.nextInt();
}
}
// 输入各进程最大需求数
System.out.println("请输入各进程最大需求数:");
for (int i = 0; i < numOfProcess; i++) {
for (int j = 0; j < numOfResource; j++) {
max[i][j] = scanner.nextInt();
need[i][j] = max[i][j] - allocation[i][j];
}
}
// 输入系统可用资源数
System.out.println("请输入系统可用资源数:");
for (int i = 0; i < numOfResource; i++) {
available[i] = scanner.nextInt();
}
}
// 判断是否存在安全序列
public boolean isSafe() {
int[] work = new int[numOfResource];
System.arraycopy(available, 0, work, 0, numOfResource); // 初始化work向量
for (int i = 0; i < numOfProcess; i++) {
if (finish[i]) {
continue;
}
boolean flag = true;
for (int j = 0; j < numOfResource; j++) {
if (need[i][j] > work[j]) {
flag = false;
break;
}
}
if (flag) {
for (int j = 0; j < numOfResource; j++) {
work[j] += allocation[i][j];
}
finish[i] = true;
i = -1; // 重新遍历
}
}
for (boolean f : finish) {
if (!f) {
return false;
}
}
return true;
}
// 输出安全序列
public void safeSequence() {
int[] work = new int[numOfResource];
int[] sequence = new int[numOfProcess];
System.arraycopy(available, 0, work, 0, numOfResource); // 初始化work向量
int count = 0;
while (count < numOfProcess) {
int i;
for (i = 0; i < numOfProcess; i++) {
if (finish[i]) {
continue;
}
boolean flag = true;
for (int j = 0; j < numOfResource; j++) {
if (need[i][j] > work[j]) {
flag = false;
break;
}
}
if (flag) {
for (int j = 0; j < numOfResource; j++) {
work[j] += allocation[i][j];
}
finish[i] = true;
sequence[count] = i;
count++;
break;
}
}
if (i == numOfProcess) { // 没有找到可以分配的进程,退出循环
break;
}
}
if (count == numOfProcess) { // 存在安全序列
System.out.print("安全序列为:");
for (int i : sequence) {
System.out.print("P" + i + " ");
}
} else { // 不存在安全序列
System.out.println("不存在安全序列!");
}
}
public static void main(String[] args) {
BankerAlgorithm banker = new BankerAlgorithm();
banker.init();
banker.safeSequence();
}
}
```
以上代码实现了银行家算法,包括输入进程已分配资源数、最大需求数和系统可用资源数,判断是否存在安全序列,输出安全序列等功能。您可以根据自己的需要进行修改和扩展。
相关推荐
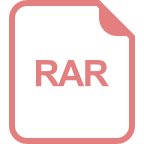
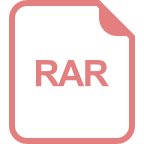
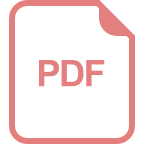













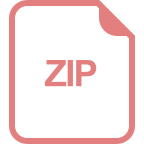
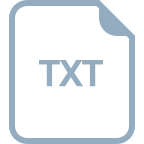