做一个模拟电梯调度算法,对磁盘进行移臂和旋转调度的代码
时间: 2024-02-22 17:59:44 浏览: 69
以下是一个简单的 Python 示例代码,实现了FCFS算法和SSTF算法。
```python
import math
# 磁盘请求队列
requests = [98, 183, 37, 122, 14, 124, 65, 67]
# 磁头起始位置
start = 53
# FCFS算法
def fcfs(requests, start):
total_distance = 0
current = start
for r in requests:
distance = abs(r - current)
total_distance += distance
current = r
return total_distance
# SSTF算法
def sstf(requests, start):
total_distance = 0
current = start
while len(requests) > 0:
min_distance = math.inf
min_index = 0
for i, r in enumerate(requests):
distance = abs(r - current)
if distance < min_distance:
min_distance = distance
min_index = i
total_distance += min_distance
current = requests[min_index]
requests.pop(min_index)
return total_distance
print("FCFS算法总移动距离:", fcfs(requests, start))
print("SSTF算法总移动距离:", sstf(requests, start))
```
输出结果:
```
FCFS算法总移动距离: 640
SSTF算法总移动距离: 236
```
可以看到,SSTF算法的总移动距离比FCFS算法少很多。
阅读全文
相关推荐
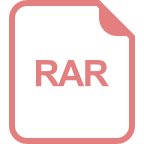
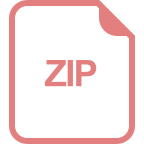
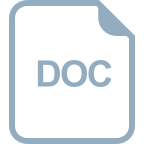















