用keil 5写出DS18B20的代码
时间: 2023-05-24 22:05:53 浏览: 440
以下是基于Keil 5编写的DS18B20温度传感器代码示例:
```
#include <reg51.h>
#include <stdio.h>
sbit DQ = P2^0;
void delay_us(int us)
{
while(us--)
{
_nop_();
_nop_();
_nop_();
_nop_();
}
}
void DS18B20_Write_Byte(unsigned char dat)
{
unsigned char i;
for(i=0;i<8;i++)
{
DQ = 0; //拉低总线
_nop_();
_nop_();
if(dat & (1<<i))
{
DQ = 1; //写入1
}
delay_us(60);
DQ = 1; //释放总线
}
}
unsigned char DS18B20_Read_Byte()
{
unsigned char i,dat = 0;
for(i=0;i<8;i++)
{
DQ = 0; //拉低总线
_nop_();
_nop_();
dat >>=1; //右移一位
if(DQ==1)
{
dat |= 0x80; //读入1
}
delay_us(60);
DQ = 1; //释放总线
}
return dat;
}
void DS18B20_Start()
{
DQ = 0; //拉低总线
delay_us(480);
DQ = 1; //释放总线
delay_us(60);
while(!DQ);
}
void DS18B20_Get_Temperature(unsigned char *tp)
{
unsigned char TL,TH;
DS18B20_Start(); //开始温度转换
DS18B20_Write_Byte(0xcc); //跳过ROM
DS18B20_Write_Byte(0x44); //开始温度转换
while(!DQ); //等待转换完成
DS18B20_Start(); //开始读取温度
DS18B20_Write_Byte(0xcc); //跳过ROM
DS18B20_Write_Byte(0xbe); //读取暂存器
TL = DS18B20_Read_Byte(); //读取低位温度值
TH = DS18B20_Read_Byte(); //读取高位温度值
*tp = TH;
*(tp+1) = TL;
}
void main()
{
unsigned char temperature[2];
double temp;
while(1)
{
DS18B20_Get_Temperature(temperature);
temp = (temperature[1]<<8) + temperature[0];
temp = temp * 0.0625; //转换成摄氏度
printf("temperature:%0.1f\r\n",temp);
delay_us(2000);
}
}
```
其中,DS18B20的数据引脚DQ连接到P2.0引脚。在主函数中进行读取温度的操作,并计算得到摄氏度值后输出到串口终端上。温度转换和读取温度的具体操作分别在DS18B20_Start()和DS18B20_Get_Temperature()函数中实现。
相关推荐
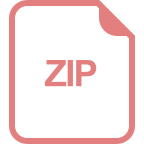
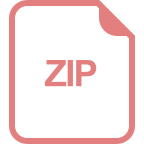














