float转为string保留两位小数 C++
时间: 2023-06-23 22:52:24 浏览: 141
你可以使用C++中的stringstream来将float转换为string并保留两位小数。以下是示例代码:
```c++
#include <iostream>
#include <iomanip>
#include <sstream>
using namespace std;
int main() {
float f = 3.14159;
stringstream s;
s << fixed << setprecision(2) << f;
string result = s.str();
cout << result << endl;
return 0;
}
```
在上面的示例中,我们使用了`fixed`和`setprecision`函数来保留两位小数,并将结果存储在stringstream对象`s`中。最后,我们使用`s.str()`函数将结果转换为string类型并打印出来。
相关问题
float 转为string保留两位小数 C++
可以使用以下代码将 float 转为 string 并保留两位小数:
```c++
#include <iostream>
#include <string>
#include <sstream>
#include <iomanip>
int main() {
float num = 3.14159;
std::ostringstream stream;
stream << std::fixed << std::setprecision(2) << num;
std::string str_num = stream.str();
std::cout << str_num << std::endl; // 输出 3.14
return 0;
}
```
这里使用了 ostringstream 类来将 float 类型的变量 num 转换为 string 类型的变量 str_num。std::fixed 表示将浮点数转换为定点数格式,std::setprecision(2) 表示保留两位小数。最后用 stream.str() 获取转换后的字符串。
float转为string并保留两位小数 C++
你可以使用 C++ 中的 `std::stringstream` 和 `std::fixed` 来实现将 `float` 转为保留两位小数的 `std::string`。具体实现如下:
```c++
#include <iostream>
#include <sstream>
#include <iomanip>
int main() {
float f = 3.1415926f;
std::stringstream stream;
stream << std::fixed << std::setprecision(2) << f;
std::string str = stream.str();
std::cout << str << std::endl;
return 0;
}
```
运行结果为:
```
3.14
```
这里 `std::fixed` 表示要使用固定点格式输出,`std::setprecision(2)` 表示保留 2 位小数。`stream.str()` 返回 `std::stringstream` 中的字符串表示。
相关推荐
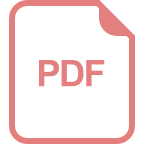
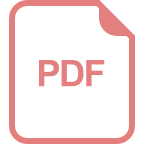












