package com.design.info.controller; import com.design.info.bean.Cate; import com.design.info.service.CateService; import com.design.common.utils.PageInfo; import com.design.common.utils.ReturnData; import com.github.pagehelper.PageHelper; import org.springframework.web.bind.annotation.*; import javax.annotation.Resource; import java.util.List; @RestController @RequestMapping("/api") @CrossOrigin(origins = "*") public class CateController { @Resource private CateService cateService; @GetMapping("/cate") public ReturnData listCate(PageInfo<?> pageInfo, Cate cate) { PageHelper.startPage(pageInfo.getCurrent(), pageInfo.getPageSize()); List<Cate> cateList = cateService.listCate(cate); return ReturnData.success().data(new PageInfo<>(cateList)); } @GetMapping("/cate/{id}") public ReturnData getCate(@PathVariable Long id) { return ReturnData.success().data(cateService.getById(id)); } @PostMapping("/cate") public ReturnData insertCate(@RequestBody Cate cate) { boolean isSuccess = cateService.save(cate); return isSuccess ? ReturnData.success() : ReturnData.error(); } @PutMapping("/cate") public ReturnData updateCate(@RequestBody Cate cate) { boolean isSuccess = cateService.updateById(cate); return isSuccess ? ReturnData.success() : ReturnData.error(); } @DeleteMapping("/cate/{id}") public ReturnData deleteCate(@PathVariable Long id) { boolean isSuccess = cateService.removeById(id); return isSuccess ? ReturnData.success() : ReturnData.error(); } }
时间: 2024-04-20 21:25:55 浏览: 107
这段代码是一个Spring Boot的控制器类,用于处理与Cate(分类)相关的请求。具体功能如下:
- `listCate`方法:接收一个`PageInfo`对象和一个`Cate`对象作为参数,使用`PageHelper`进行分页查询,调用`cateService.listCate(cate)`方法获取分类列表,并将结果封装到`PageInfo`对象中返回。
- `getCate`方法:根据传入的`id`参数调用`cateService.getById(id)`方法获取指定id的分类信息,并将结果封装到`ReturnData`对象中返回。
- `insertCate`方法:接收一个`Cate`对象作为参数,调用`cateService.save(cate)`方法保存分类信息,并根据保存结果返回相应的`ReturnData`对象。
- `updateCate`方法:接收一个`Cate`对象作为参数,调用`cateService.updateById(cate)`方法更新分类信息,并根据更新结果返回相应的`ReturnData`对象。
- `deleteCate`方法:根据传入的`id`参数调用`cateService.removeById(id)`方法删除指定id的分类信息,并根据删除结果返回相应的`ReturnData`对象。
该控制器类使用了`@RestController`注解标识为控制器,并通过`@RequestMapping("/api")`设置了基础请求路径为"/api"。同时使用了`@CrossOrigin(origins = "*")`注解开启跨域访问。
相关问题
package com.design.info.service; import com.baomidou.mybatisplus.core.toolkit.StringUtils; import com.baomidou.mybatisplus.core.toolkit.Wrappers; import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl; import com.design.info.bean.Cate; import com.design.info.dao.CateMapper; import org.springframework.stereotype.Service; import javax.annotation.Resource; import java.util.List; @Service public class CateService extends ServiceImpl<CateMapper, Cate> { @Resource private CateMapper cateMapper; public List<Cate> listCate(Cate cate) { return cateMapper.selectList(Wrappers.<Cate>lambdaQuery() .like(StringUtils.isNotBlank(cate.getName()), Cate::getName, cate.getName()) .eq(cate.getActive() != null, Cate::getActive, cate.getActive()) .orderByAsc(Cate::getSort) ); } }
这段代码是一个使用了 MyBatis Plus 的 Service 层示例,继承了 `ServiceImpl<CateMapper, Cate>` 类,该类提供了一些常用的数据库操作方法的实现。
在该示例中,`CateService` 类使用了 `@Service` 注解来标识它是一个 Service 类,并注入了 `CateMapper` 对象来进行数据库操作。
其中,`listCate()` 方法用于查询符合条件的分类列表。在该方法中,使用了 MyBatis Plus 提供的 `Wrappers.<Cate>lambdaQuery()` 来创建查询条件构造器,使用了链式调用的方式进行条件拼接。
- `like(StringUtils.isNotBlank(cate.getName()), Cate::getName, cate.getName())`:当传入的 `cate` 对象的 `name` 字段不为空时,使用 `like` 条件进行模糊查询。
- `eq(cate.getActive() != null, Cate::getActive, cate.getActive())`:当传入的 `cate` 对象的 `active` 字段不为 null 时,使用 `eq` 条件进行等值查询。
- `orderByAsc(Cate::getSort)`:按照分类的 `sort` 字段进行升序排序。
最后,通过调用 `cateMapper.selectList()` 方法来执行查询操作,并返回查询结果列表。
希望对您有所帮助!如果您还有其他问题,请随时提问。
package com.design.info.dao; import com.baomidou.mybatisplus.core.mapper.BaseMapper; import com.design.info.bean.Cate; import org.apache.ibatis.annotations.Mapper; @Mapper public interface CateMapper extends BaseMapper<Cate> { }
这段代码是一个使用 MyBatis Plus 的 Mapper 接口示例,用于操作数据库表对应的实体类 Cate。
- `@Mapper` 注解表示该接口是一个 MyBatis 的 Mapper 接口,用于与数据库进行交互。
- `extends BaseMapper<Cate>` 表示该接口继承自 `BaseMapper` 接口,并指定泛型为实体类 Cate。通过继承 `BaseMapper` 接口,可以获得一些常用的数据库操作方法,如插入、更新、删除和查询等。
该接口中并没有自定义的方法,但可以直接使用 `BaseMapper` 提供的方法来进行数据库操作。例如,可以使用 `insert()` 方法插入数据,使用 `updateById()` 方法根据主键更新数据,使用 `deleteById()` 方法根据主键删除数据,使用 `selectById()` 方法根据主键查询数据等。
使用该接口时,需要在 Spring Boot 的配置文件中配置 MyBatis Plus 的扫描路径,让其能够自动识别并注入该 Mapper 接口。
希望对您有所帮助!如有更多疑问,请随时提问。
阅读全文
相关推荐
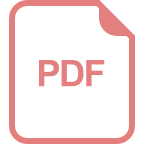
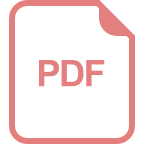
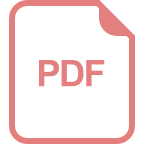
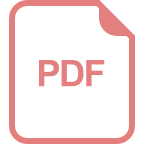













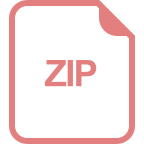