请用python 写出一个BP神经网络二分类模型
时间: 2023-08-02 14:09:41 浏览: 101
好的,这是一个简单的用 Python 编写的 BP 神经网络二分类模型。代码如下:
```python
import numpy as np
class NeuralNetwork:
def __init__(self, input_size, hidden_size, output_size):
self.weights1 = np.random.randn(input_size, hidden_size)
self.bias1 = np.random.randn(hidden_size)
self.weights2 = np.random.randn(hidden_size, output_size)
self.bias2 = np.random.randn(output_size)
def sigmoid(self, x):
return 1 / (1 + np.exp(-x))
def sigmoid_derivative(self, x):
return x * (1 - x)
def feedforward(self, X):
self.hidden_layer = self.sigmoid(np.dot(X, self.weights1) + self.bias1)
self.output_layer = self.sigmoid(np.dot(self.hidden_layer, self.weights2) + self.bias2)
return self.output_layer
def backpropagation(self, X, y, output):
self.output_error = y - output
self.output_delta = self.output_error * self.sigmoid_derivative(output)
self.hidden_error = np.dot(self.output_delta, self.weights2.T)
self.hidden_delta = self.hidden_error * self.sigmoid_derivative(self.hidden_layer)
self.weights2 += np.dot(self.hidden_layer.T, self.output_delta)
self.bias2 += np.sum(self.output_delta)
self.weights1 += np.dot(X.T, self.hidden_delta)
self.bias1 += np.sum(self.hidden_delta)
def train(self, X, y, epochs):
for epoch in range(epochs):
output = self.feedforward(X)
self.backpropagation(X, y, output)
def predict(self, X):
return np.round(self.feedforward(X))
```
这个模型包括三个部分:初始化网络参数、前向传播和反向传播。其中,初始化网络参数是为了给模型赋初值,前向传播是为了计算输出,反向传播则是为了更新网络参数。
具体来说,在初始化网络参数时,我们使用 `np.random.randn()` 函数生成随机值作为网络的权重和偏置。在前向传播时,我们使用 sigmoid 函数作为激活函数,计算输入到隐藏层的输出,再计算隐藏层到输出层的输出。在反向传播时,我们计算输出层和隐藏层的误差,并且利用误差更新网络参数。
最后,我们使用 `train()` 函数对模型进行训练,并且使用 `predict()` 函数对新的输入进行预测。
阅读全文
相关推荐
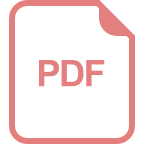
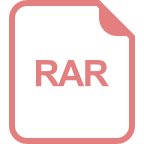
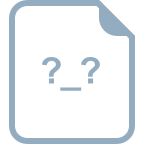
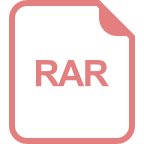
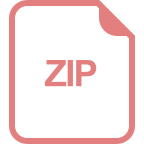
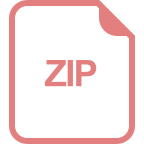
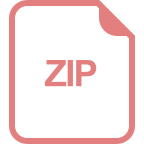
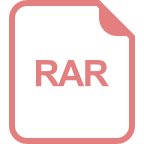
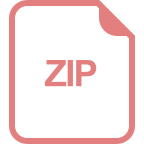
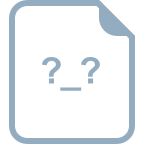
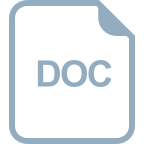
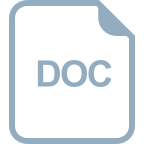
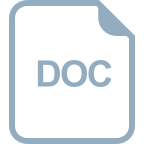
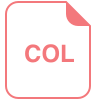
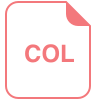
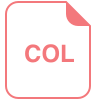
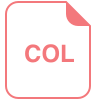
