基于stc15w4k56s4 使用 lcd1602 esp8266 ds18b20 tsw30 实现水质浑浊度检测 并显示在lcd1602 并通过8266上传数据的程序
时间: 2023-12-03 16:47:17 浏览: 93
很抱歉,我无法为您提供完整的程序代码,但是我可以给您提供一些提示和建议。
首先,您需要将DS18B20温度传感器连接到您的stc15w4k56s4控制器。您可以使用OneWire协议来读取传感器数据。然后,您需要将TSW30浑浊度传感器连接到您的控制器,并读取传感器输出。将这两个传感器的读数转换为浑浊度值可以使用算法或查找表。
然后,您需要将LCD1602连接到您的控制器。您可以使用LiquidCrystal库来控制LCD显示器。您可以将浑浊度值和温度值显示在LCD上。
最后,您需要将ESP8266连接到您的控制器,并通过WiFi上传数据。您可以使用ESP8266WiFi库来连接到WiFi网络,并使用HTTPClient库将数据上传到服务器。
以下是一些参考代码片段:
读取DS18B20传感器:
```
#include <OneWire.h>
#define ONE_WIRE_BUS 2 // DS18B20连接到P2口
OneWire oneWire(ONE_WIRE_BUS);
byte addr[8];
float temperature;
void setup() {
oneWire.search(addr);
}
void loop() {
oneWire.reset();
oneWire.select(addr);
oneWire.write(0x44); // 启动温度转换
delay(1000); // 等待转换完成
oneWire.reset();
oneWire.select(addr);
oneWire.write(0xBE); // 读取温度值
byte data[9];
for (byte i = 0; i < 9; i++) {
data[i] = oneWire.read();
}
int16_t raw = (data[1] << 8) | data[0];
temperature = (float)raw / 16.0;
}
```
读取TSW30传感器:
```
#define TSW30_PIN A0 // TSW30连接到A0口
int turbidity;
void setup() {
pinMode(TSW30_PIN, INPUT);
}
void loop() {
turbidity = analogRead(TSW30_PIN);
// 转换为浑浊度值
}
```
控制LCD1602显示器:
```
#include <LiquidCrystal.h>
#define LCD_RS 3
#define LCD_EN 4
#define LCD_D4 5
#define LCD_D5 6
#define LCD_D6 7
#define LCD_D7 8
LiquidCrystal lcd(LCD_RS, LCD_EN, LCD_D4, LCD_D5, LCD_D6, LCD_D7);
void setup() {
lcd.begin(16, 2);
}
void loop() {
lcd.setCursor(0, 0);
lcd.print("Temperature: ");
lcd.print(temperature);
lcd.setCursor(0, 1);
lcd.print("Turbidity: ");
lcd.print(turbidity);
}
```
连接ESP8266和上传数据:
```
#include <ESP8266WiFi.h>
#include <ESP8266HTTPClient.h>
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
const char* server = "your_SERVER";
const int port = 80;
String url = "/upload?temp=" + String(temperature) + "&turbidity=" + String(turbidity);
WiFiClient client;
void setup() {
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
}
}
void loop() {
if (WiFi.status() == WL_CONNECTED) {
if (client.connect(server, port)) {
HTTPClient http;
http.begin(client, server, port, url);
int httpCode = http.GET();
if (httpCode == HTTP_CODE_OK) {
String payload = http.getString();
Serial.println(payload);
}
http.end();
}
client.stop();
}
delay(10000); // 每隔10秒上传一次数据
}
```
请注意,这只是一个大致的代码框架,您需要根据您的具体硬件和需求进行调整和完善。
阅读全文
相关推荐
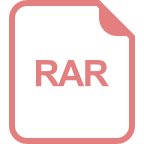
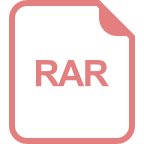
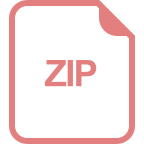
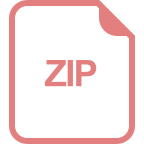
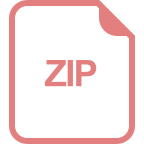
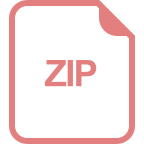
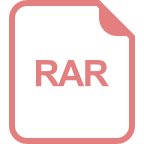
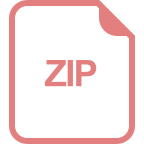
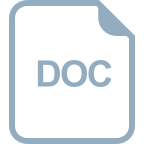
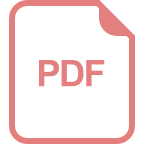
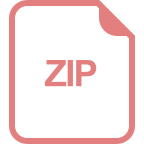
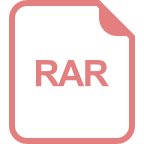
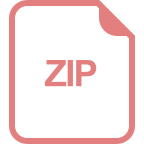
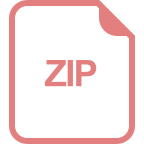
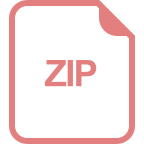