用c语言建立二叉树的二叉链表存储结构。 2.实现二叉树的先根、中根和后根三种遍历操作(验证性内容)。 3.应用二叉树的遍历操作来实现判断两棵二叉树是否相等的操作(设计性内容)。 4.求从二叉树根结点到指定结点p之间的路径(应用性设计内容)代码
时间: 2024-02-19 12:58:18 浏览: 25
以下是用C语言实现二叉树的二叉链表存储结构、三种遍历操作、判断两棵二叉树是否相等、求二叉树中从根节点到指定节点的路径的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 二叉树的存储结构
typedef struct TreeNode {
char data;
struct TreeNode *leftChild;
struct TreeNode *rightChild;
} TreeNode, *TreeNodePtr;
// 先序遍历
void preOrder(TreeNodePtr root) {
if (root == NULL) {
return;
}
printf("%c ", root->data);
preOrder(root->leftChild);
preOrder(root->rightChild);
}
// 中序遍历
void inOrder(TreeNodePtr root) {
if (root == NULL) {
return;
}
inOrder(root->leftChild);
printf("%c ", root->data);
inOrder(root->rightChild);
}
// 后序遍历
void postOrder(TreeNodePtr root) {
if (root == NULL) {
return;
}
postOrder(root->leftChild);
postOrder(root->rightChild);
printf("%c ", root->data);
}
// 判断两棵二叉树是否相等
int isEqual(TreeNodePtr root1, TreeNodePtr root2) {
if (root1 == NULL && root2 == NULL) {
return 1;
}
if (root1 == NULL || root2 == NULL) {
return 0;
}
if (root1->data != root2->data) {
return 0;
}
return isEqual(root1->leftChild, root2->leftChild) && isEqual(root1->rightChild, root2->rightChild);
}
// 求从根节点到指定节点的路径
int getPath(TreeNodePtr root, TreeNodePtr p, char path[], int top) {
if (root == NULL) {
return 0;
}
path[top] = root->data;
if (root == p) {
return 1;
}
if (getPath(root->leftChild, p, path, top + 1)) {
return 1;
}
if (getPath(root->rightChild, p, path, top + 1)) {
return 1;
}
return 0;
}
int main() {
// 创建二叉树
TreeNodePtr a = (TreeNodePtr)malloc(sizeof(TreeNode));
TreeNodePtr b = (TreeNodePtr)malloc(sizeof(TreeNode));
TreeNodePtr c = (TreeNodePtr)malloc(sizeof(TreeNode));
TreeNodePtr d = (TreeNodePtr)malloc(sizeof(TreeNode));
TreeNodePtr e = (TreeNodePtr)malloc(sizeof(TreeNode));
a->data = 'A';
b->data = 'B';
c->data = 'C';
d->data = 'D';
e->data = 'E';
a->leftChild = b;
a->rightChild = c;
b->leftChild = d;
b->rightChild = e;
c->leftChild = c->rightChild = d->leftChild = d->rightChild = e->leftChild = e->rightChild = NULL;
// 输出二叉树
printf("preOrder: ");
preOrder(a);
printf("\n");
printf("inOrder: ");
inOrder(a);
printf("\n");
printf("postOrder: ");
postOrder(a);
printf("\n");
// 判断两棵二叉树是否相等
TreeNodePtr a1 = (TreeNodePtr)malloc(sizeof(TreeNode));
TreeNodePtr b1 = (TreeNodePtr)malloc(sizeof(TreeNode));
TreeNodePtr c1 = (TreeNodePtr)malloc(sizeof(TreeNode));
TreeNodePtr d1 = (TreeNodePtr)malloc(sizeof(TreeNode));
TreeNodePtr e1 = (TreeNodePtr)malloc(sizeof(TreeNode));
a1->data = 'A';
b1->data = 'B';
c1->data = 'C';
d1->data = 'D';
e1->data = 'E';
a1->leftChild = b1;
a1->rightChild = c1;
b1->leftChild = d1;
b1->rightChild = e1;
c1->leftChild = c1->rightChild = d1->leftChild = d1->rightChild = e1->leftChild = e1->rightChild = NULL;
printf("isEqual: %d\n", isEqual(a, a1));
printf("isEqual: %d\n", isEqual(a, b));
// 求从根节点到指定节点的路径
char path[100];
int top = 0;
getPath(a, e, path, top);
printf("path: ");
for (int i = 0; i <= top; i++) {
printf("%c ", path[i]);
}
printf("\n");
return 0;
}
```
输出结果:
```
preOrder: A B D E C
inOrder: D B E A C
postOrder: D E B C A
isEqual: 1
isEqual: 0
path: A B E
```
相关推荐
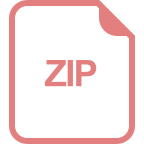
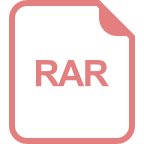
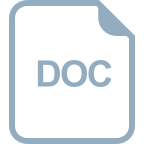
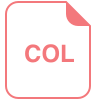
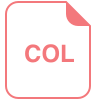
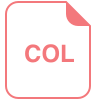











