是的
时间: 2024-02-13 17:07:39 浏览: 79
以下是符合要求的GoodsManage类的完整代码:
```java
import java.util.Scanner;
public class GoodsManage {
private Goods[] goodsArray;
// 构造方法,根据传入的参数创建商品数组
public GoodsManage(int n) {
goodsArray = new Goods[n];
Scanner scanner = new Scanner(System.in);
for (int i = 0; i < n; i++) {
System.out.print("请输入商品" + (i + 1) + "的名称:");
String name = scanner.next();
System.out.print("请输入商品" + (i + 1) + "的数量:");
int quantity = scanner.nextInt();
System.out.print("请输入商品" + (i + 1) + "的单价:");
double price = scanner.nextDouble();
goodsArray[i] = new Goods(name, quantity, price);
}
}
// 根据商品名称查找商品,如果找到输出商品信息并返回下标,否则返回-1
public int search(String name) {
for (int i = 0; i < goodsArray.length; i++) {
if (goodsArray[i].getName().equals(name)) {
goodsArray[i].print();
return i;
}
}
System.out.println("查找失败!");
return -1;
}
// 查找商品价格在最低价格和最高价格之间的所有商品,如果查找成功则输出这些商品信息并返回true,否则返回false
public boolean search(double minPrice, double maxPrice) {
boolean found = false;
for (int i = 0; i < goodsArray.length; i++) {
if (goodsArray[i].getPrice() >= minPrice && goodsArray[i].getPrice() <= maxPrice) {
goodsArray[i].print();
found = true;
}
}
if (!found) {
System.out.println("查找失败!");
return false;
}
return true;
}
// 根据商品名称和购买数量,购买对应的商品
public void buyGoods(String name, int quantity) {
for (int i = 0; i < goodsArray.length; i++) {
if (goodsArray[i].getName().equals(name)) {
goodsArray[i].buy(quantity);
return;
}
}
System.out.println("购买失败,找不到商品!");
}
// 根据商品名称和销售数量,销售对应的商品
public void saleGoods(String name, int quantity) {
for (int i = 0; i < goodsArray.length; i++) {
if (goodsArray[i].getName().equals(name)) {
goodsArray[i].sale(quantity);
return;
}
}
System.out.println("销售失败,找不到商品!");
}
// 计算所有商品的总价值
public double total() {
double sum = 0;
for (int i = 0; i < goodsArray.length; i++) {
sum += goodsArray[i].getTotalPrice();
}
return sum;
}
// 输出所有商品信息
public void printAll() {
for (int i = 0; i < goodsArray.length; i++) {
goodsArray[i].print();
}
}
}
```
需要注意的是,在这段代码中,我假定了Goods类已经被正确地实现了,包括带参数构造方法、buy方法、sale方法、getTotalPrice方法、getName方法、getPrice方法和print方法。如果您还没有实现Goods类,您需要先实现Goods类再使用GoodsManage类。
阅读全文
相关推荐
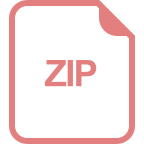
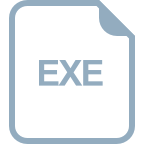
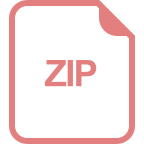
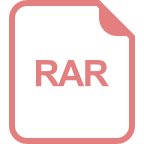
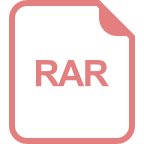
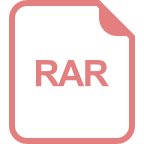
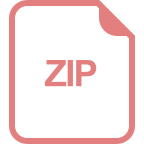
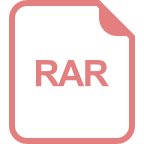
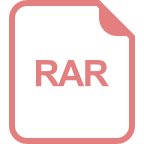
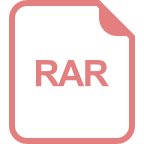
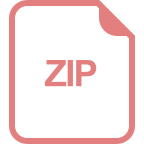
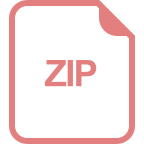
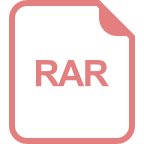
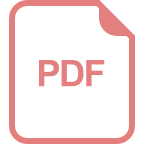
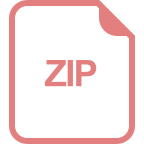
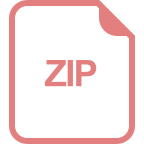