使用Java实现银行家算法
时间: 2023-11-16 11:07:09 浏览: 62
银行家算法是一种避免死锁的算法,主要用于操作系统中的资源分配。下面是使用Java实现银行家算法的代码示例:
```java
import java.util.Scanner;
public class BankerAlgorithm {
private int[][] need;
private int[][] allocation;
private int[][] max;
private int[] available;
private int numOfProcesses;
private int numOfResources;
public BankerAlgorithm() {
Scanner sc = new Scanner(System.in);
System.out.print("请输入进程数:");
numOfProcesses = sc.nextInt();
System.out.print("请输入资源数:");
numOfResources = sc.nextInt();
need = new int[numOfProcesses][numOfResources];
allocation = new int[numOfProcesses][numOfResources];
max = new int[numOfProcesses][numOfResources];
available = new int[numOfResources];
System.out.println("请输入各进程的最大需求量:");
for (int i = 0; i < numOfProcesses; i++) {
for (int j = 0; j < numOfResources; j++) {
max[i][j] = sc.nextInt();
}
}
System.out.println("请输入各进程的已分配量:");
for (int i = 0; i < numOfProcesses; i++) {
for (int j = 0; j < numOfResources; j++) {
allocation[i][j] = sc.nextInt();
need[i][j] = max[i][j] - allocation[i][j];
}
}
System.out.println("请输入各资源的总数:");
for (int i = 0; i < numOfResources; i++) {
available[i] = sc.nextInt();
}
}
private boolean isSafe() {
int[] work = new int[numOfResources];
int[] finish = new int[numOfProcesses];
for (int i = 0; i < numOfResources; i++) {
work[i] = available[i];
}
int count = 0;
while (count < numOfProcesses) {
boolean found = false;
for (int i = 0; i < numOfProcesses; i++) {
if (finish[i] == 0) {
int j;
for (j = 0; j < numOfResources; j++) {
if (need[i][j] > work[j])
break;
}
if (j == numOfResources) {
for (int k = 0; k < numOfResources; k++) {
work[k] += allocation[i][k];
}
finish[i] = 1;
found = true;
count++;
}
}
}
if (!found) {
return false;
}
}
return true;
}
public void run() {
if (isSafe()) {
System.out.println("系统是安全的!");
} else {
System.out.println("系统是不安全的!");
}
}
public static void main(String[] args) {
BankerAlgorithm bankerAlgorithm = new BankerAlgorithm();
bankerAlgorithm.run();
}
}
```
在该实现中,首先输入进程数和资源数,然后输入各进程的最大需求量、已分配量和各资源的总数。接着,通过 `isSafe()` 方法检查系统是否安全,如果安全则输出“系统是安全的!”否则输出“系统是不安全的!”。
其中,`isSafe()` 方法实现了银行家算法的过程。该方法首先初始化工作向量 `work` 和完成向量 `finish`,并将工作向量初始化为可用资源向量 `available`。然后,使用循环遍历所有进程,如果该进程未完成,且它所需的每个资源都小于或等于工作向量中对应的资源,则将该进程添加到完成向量中,并更新工作向量。如果找不到满足条件的进程,则说明系统不安全。
最后,在 `main()` 方法中创建 `BankerAlgorithm` 对象并调用 `run()` 方法来执行程序。
阅读全文
相关推荐
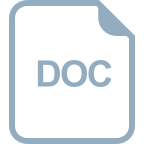
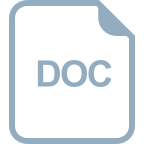
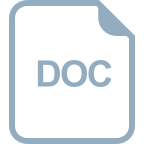
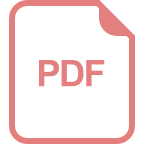

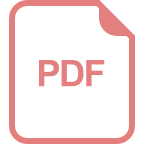
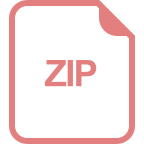
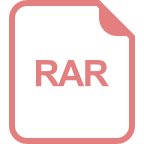
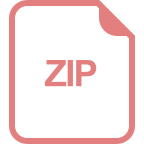


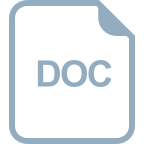
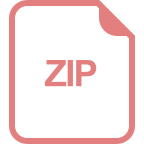
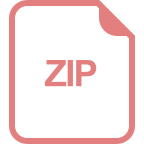
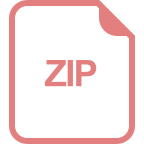
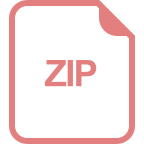
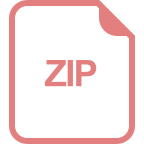
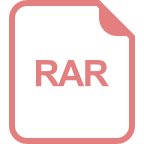