TypeError: unsupported operand type(s) for %: 'type' and 'int'
时间: 2023-07-30 16:07:13 浏览: 76
这个错误通常表示你在使用字符串格式化时,格式字符串和参数类型不匹配。请检查你的格式字符串和参数类型是否匹配。例如,如果你要将一个整数格式化为字符串,你可以使用以下代码:
```
num = 42
str_num = "The answer is %d" % num
print(str_num)
```
如果你要将一个浮点数格式化为字符串,你可以使用以下代码:
```
pi = 3.1415926
str_pi = "Pi is approximately %.2f" % pi
print(str_pi)
```
在这个例子中,%.2f 表示将浮点数格式化为小数点后两位的字符串。
相关问题
TypeError: unsupported operand type(s) for %: 'int' and 'str'
遇到`TypeError: unsupported operand type(s) for %: 'int' and 'str'`错误时,意味着您尝试对整数(int)和字符串(str)执行不兼容的操作,这里是%操作符(模运算符),通常用于格式化字符串。
在Python中,当你试图使用`%`来格式化一个字符串,其中一个操作数是整数,另一个是字符串,就会引发这个错误,因为字符串不能直接与数字做算术运算。
解决这个问题的方法有几种:
1. **确保两边都是可格式化的对象**:如果想在字符串中插入变量,确保那个变量已经是字符串形式。比如,如果你有一个整数 `num` 和一个你想格式化的字符串 `"My number is {}."`,你应该先将 `num` 转换成字符串,然后再格式化:
```python
num = 42
formatted_str = "My number is {}.".format(str(num))
```
2. **使用f-string (格式化字符串)**:Python 3.6及以上版本引入了更简洁的字符串格式化方式,可以直接在字符串中嵌入表达式:
```python
num = 42
formatted_str = f"My number is {num}."
```
3. **转换字符串**:如果一定要使用`%`运算符,可以先将字符串转成整数再进行计算,但这不是推荐的做法,因为它可能引发其他问题:
```python
num = 42
str_num = str(num)
formatted_str = "My number is %s." % str_num
```
xlsxwriter写入时TypeError: unsupported operand type(s) for %: ‘int’ and ‘str’
当遇到`TypeError: unsupported operand type(s) for %: 'int' and 'str'`错误时,这意味着你在尝试使用百分比运算符(%)对一个整数和一个字符串进行操作,这在Python中是不允许的。在xlsxwriter库中,可能是在试图将一个整数格式化为字符串以便于与单元格内容结合使用时发生的。
要解决这个问题,你需要确保在使用百分比运算之前,将整数转换为字符串。这里有一个简单的示例:
```python
from xlsxwriter import Workbook
# 假设你有整数total和要写入的百分比value
total = 100
value = 0.5
# 先将value转换为字符串
percentage_value = '{:.2%}'.format(value)
workbook = Workbook('output.xlsx')
worksheet = workbook.add_worksheet()
# 写入单元格,注意这里的percent替换为已经转换的percentage_value
worksheet.write('A1', total)
worksheet.write('B1', "Percentage: " + percentage_value)
workbook.close()
```
这样,你会得到一个格式化的百分比,如"50.00%",而不会引发`TypeError`。
阅读全文
相关推荐
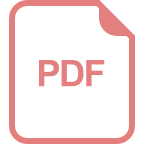
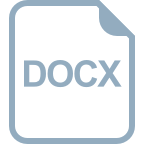
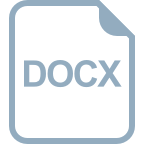













