c#udp
时间: 2023-07-10 16:25:44 浏览: 111
C#中的UDP是指User Datagram Protocol(用户数据报协议),是一种无连接的协议,它不需要在发送数据前先建立连接。在C#中,可以使用UdpClient类来实现UDP通信。使用UdpClient类,可以发送和接收UDP数据报。以下是一个简单的示例代码,用于发送UDP数据报:
```csharp
using System;
using System.Net;
using System.Net.Sockets;
class Program
{
static void Main(string[] args)
{
// 创建一个UdpClient对象
UdpClient udpClient = new UdpClient();
// 设置目标IP地址和端口号
IPAddress ipAddress = IPAddress.Parse("192.168.1.100");
int port = 1234;
// 将要发送的数据转换为字节数组
string message = "Hello, World!";
byte[] sendBytes = Encoding.ASCII.GetBytes(message);
// 发送数据报
udpClient.Send(sendBytes, sendBytes.Length, new IPEndPoint(ipAddress, port));
// 关闭UdpClient对象
udpClient.Close();
}
}
```
接收UDP数据报的示例代码如下:
```csharp
using System;
using System.Net;
using System.Net.Sockets;
class Program
{
static void Main(string[] args)
{
// 创建一个UdpClient对象,并绑定到本地IP地址和端口号
UdpClient udpClient = new UdpClient(1234);
// 接收数据报
IPEndPoint remoteEndPoint = new IPEndPoint(IPAddress.Any, 0);
byte[] receiveBytes = udpClient.Receive(ref remoteEndPoint);
// 将接收到的字节数组转换为字符串
string message = Encoding.ASCII.GetString(receiveBytes);
// 输出接收到的数据
Console.WriteLine("Received: {0} from {1}", message, remoteEndPoint);
// 关闭UdpClient对象
udpClient.Close();
}
}
```
阅读全文
相关推荐



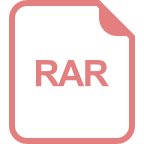








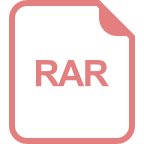


