#include <stdio.h> typedef struct { int year; float salary; } Data;//定义数据结构体,存储年份和平均工资的数据 int main(int argc, char* argv[]) { // Sample data Data sample_data[] = { {2012, 3450}, {2013, 3785}, {2014, 4380}, {2015, 4580}, {2017, 5425}, {2018, 6060}, {2019, 6320}, {2020, 6880}, {2021, 7120}, {2022, 7360}, }; int sample_size = sizeof(sample_data) / sizeof(Data); //定义样本数据数组,并初始化 // 开始绘制散点图 FILE* gp = _popen("gnuplot -persist", "w"); fprintf(gp, "set title 'Average Salary of Graduates'\n"); fprintf(gp, "set xlabel 'Year'\n"); fprintf(gp, "set ylabel 'Salary'\n"); fprintf(gp, "plot '-' with points pointtype 6 pointsize 1.5 title 'Sample Data'\n"); for (int i = 0; i < sample_size; i++) { fprintf(gp, "%d %f\n", sample_data[i].year, sample_data[i].salary); } fprintf(gp, "e\n"); fflush(gp); // 使用最小二乘法拟合曲线 float sx = 0, sy = 0, sxx = 0, sxy = 0; for (int i = 0; i < sample_size; i++) { sx += sample_data[i].year; sy += sample_data[i].salary; sxx += sample_data[i].year * sample_data[i].year; sxy += sample_data[i].year * sample_data[i].salary; } float a = (sxy * sample_size - sx * sy) / (sxx * sample_size - sx * sx); float b = (sxx * sy - sx * sxy) / (sxx * sample_size - sx * sx); // 将拟合曲线绘制到散点图上 fprintf(gp, "set xrange [%d:%d]\n", sample_data[0].year, sample_data[sample_size - 1].year + 1); fprintf(gp, "f(x) = %f * x + %f\n", a, b); fprintf(gp, "plot '-' with points pointtype 6 pointsize 1.5 title 'Sample Data', f(x) with lines linewidth 2 title 'Fitted Curve'\n"); for (int i = 0; i < sample_size; i++) { fprintf(gp, "%d %f\n", sample_data[i].year, sample_data[i].salary); } fprintf(gp, "e\n"); fflush(gp); // 预测明年的平均工资 //int next_year = sample_data[sample_size - 1].year + 1; //float next_salary = a * next_year + b; //printf("预计 %d 年毕业生的平均工资是 %.2f\n", next_year, next
时间: 2024-01-05 19:03:35 浏览: 81
_salary); // 关闭 gnuplot 进程 _pclose(gp); return 0; }
这是一个使用最小二乘法拟合散点图并预测下一年数据的 C 语言程序。它先给出了一组样本数据(年份和平均工资),然后使用 gnuplot 绘制出散点图,接着使用最小二乘法计算出拟合曲线的系数,再将拟合曲线绘制到散点图上。最后,还可以预测出下一年的平均工资。
相关问题
#include<stdio.h> #include<malloc.h> typedef struct { int no; char name[10]; int depno; float salary; }EmpType; typedef struct LNode{ EmpType date; struct LNode*next; }LinkNode;改错
这段代码本身没有错误,但是缺少了一些必要的部分,比如创建链表、插入节点等操作的函数。同时,还需要注意以下几个问题:
1. 在使用 typedef 定义结构体时,结构体名和别名应该分别写在 struct 和 typedef 后面,例如:
```
typedef struct EmpType {
int no;
char name[10];
int depno;
float salary;
} EmpType;
```
2. 在定义结构体指针成员时,应该使用箭头符号 -> 而不是点号 .,例如:
```
LinkNode *p;
p->date.no = 1001;
```
3. 在使用 malloc 函数分配内存时,应该根据需要分配的内存大小来确定参数,例如:
```
LinkNode *p = (LinkNode*)malloc(sizeof(LinkNode));
```
4. 在定义结构体数组时,应该使用大括号 {} 而不是小括号 (),例如:
```
EmpType emp[10] = {
{1001, "John", 1, 5000},
{1002, "Mary", 2, 6000},
...
};
```
定义一个包含5个教师成员的结构体数组,教师信息包括职工号、姓名和工资。要求从键盘输入所有教师的信息,并设计一个sort函数实现结构体数组按教师工资由少到多排序。目前,已编辑完成main函数,请编程实现input函数、output函数和sort函数,分别实现教师信息的录入、输出与排序。 /* @Filename: ex701.c @Author: Ju Chengdong @Version: 1.0 @Date: 2021-03-18 @Description: Salary Management System */ #include <stdio.h> #include <string.h> typedef struct teacher{ int id; // 职工号 char name[100]; // 姓名 int salary; // 工资 }teacher; const int NUM = 5; int main(){ void input(teacher *t, int n); void output(teacher *t, int n); void sort(teacher *t, int n); teacher t[NUM]; //教师数组 teacher *pt = t; input(pt, NUM); //为教师数组元素赋值 printf("Before sorting:\n"); output(pt, NUM); //排序前输出 sort(pt, NUM); //按工资排序 printf("After sorting:\n"); output(pt, NUM); //排序后输出 return 0; }
好的,以下是实现代码:
```c
#include <stdio.h>
#include <string.h>
typedef struct teacher {
int id; // 职工号
char name[100]; // 姓名
int salary; // 工资
} teacher;
void input(teacher *t, int n) {
for(int i = 0; i < n; i++) {
printf("请输入第%d个教师的职工号、姓名和工资(用空格分开):", i+1);
scanf("%d %s %d", &(t+i)->id, (t+i)->name, &(t+i)->salary);
}
}
void output(teacher *t, int n) {
printf("职工号\t姓名\t工资\n");
for(int i = 0; i < n; i++) {
printf("%d\t%s\t%d\n", (t+i)->id, (t+i)->name, (t+i)->salary);
}
}
void sort(teacher *t, int n) {
teacher temp;
for(int i = 0; i < n-1; i++) {
for(int j = 0; j < n-i-1; j++) {
if((t+j)->salary > (t+j+1)->salary) {
temp = *(t+j);
*(t+j) = *(t+j+1);
*(t+j+1) = temp;
}
}
}
}
int main() {
teacher t[NUM]; //教师数组
teacher *pt = t;
input(pt, NUM); //为教师数组元素赋值
printf("Before sorting:\n");
output(pt, NUM); //排序前输出
sort(pt, NUM); //按工资排序
printf("After sorting:\n");
output(pt, NUM); //排序后输出
return 0;
}
```
在这个代码中,我们同样定义了一个结构体`teacher`,包含了三个成员变量:职工号、姓名和工资。然后我们输入了所有教师的信息,并将其存储在一个结构体数组中。最后,我们使用冒泡排序对结构体数组进行排序,排序的方式是按照教师工资由少到多排序。最后,我们输出排序后的结果。
阅读全文
相关推荐
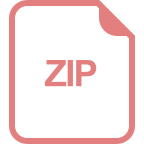
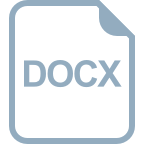
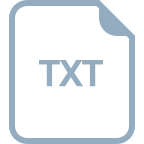













