编写html脚本,创建Vue实例,在实例中显示个人信息及成绩信息,并进行简单的交互。具体功能要求如下。 1.初始显示本人的基本信息及所有课程的成绩信息,通过data属性设置,具体信息内容如下。 (1)基本信息:姓名、学号、性别、出生日期、籍贯等,可以根据情况增加本人相关的其他信息。 (2)上学期的所有课程的成绩信息,包括:课程编码、课程名称、课程性质、学分、成绩等。 (3)所有个人信息及成绩信息必须真实。 2.每门课程的成绩添加两个按钮“加分”、“减分”,点击之后相应该课程的成绩随机增加或减少1至10分,通过元素事件绑定实例中的methods方法实现。 3.每门课程计算其绩点和学分绩点,通过methods方法实现。 4.添加相关computed属性,计算所有课程的总分、平均分。 5.监听“加分”或“减分”的次数,若次数超过2至4之间的一个随机数,则每一门课程的成绩减去10分,且“加分”、“减分”点击时,分数不再变化。 6.页面选用恰当元素,页面布局合理美观。
时间: 2023-06-16 12:06:48 浏览: 140
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>个人信息及成绩信息</title>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
</head>
<body>
<div id="app">
<h2>{{ name }}</h2>
<p>学号:{{ studentId }}</p>
<p>性别:{{ gender }}</p>
<p>出生日期:{{ birthday }}</p>
<p>籍贯:{{ hometown }}</p>
<hr>
<table>
<tr>
<th>课程编码</th>
<th>课程名称</th>
<th>课程性质</th>
<th>学分</th>
<th>成绩</th>
<th>操作</th>
</tr>
<tr v-for="(course, index) in courses" :key="index">
<td>{{ course.code }}</td>
<td>{{ course.name }}</td>
<td>{{ course.property }}</td>
<td>{{ course.credit }}</td>
<td>{{ course.score }}</td>
<td>
<button @click="addScore(index)">加分</button>
<button @click="minusScore(index)">减分</button>
</td>
</tr>
<tr>
<td colspan="4">总分:</td>
<td>{{ totalScore }}</td>
</tr>
<tr>
<td colspan="4">平均分:</td>
<td>{{ averageScore }}</td>
</tr>
</table>
</div>
<script>
var app = new Vue({
el: '#app',
data: {
name: '张三',
studentId: '20190001',
gender: '男',
birthday: '2000-01-01',
hometown: '北京市',
courses: [
{
code: '001',
name: '高等数学',
property: '必修',
credit: 4,
score: 85
},
{
code: '002',
name: '英语',
property: '必修',
credit: 2,
score: 90
},
{
code: '003',
name: '计算机科学导论',
property: '必修',
credit: 3,
score: 95
},
{
code: '004',
name: '线性代数',
property: '选修',
credit: 3,
score: 80
},
{
code: '005',
name: '数据结构',
property: '选修',
credit: 4,
score: 88
},
],
addCount: [0, 0, 0, 0, 0],
minusCount: [0, 0, 0, 0, 0]
},
methods: {
addScore: function(index) {
if (this.addCount[index] < 2) {
this.courses[index].score += Math.floor(Math.random() * 10) + 1;
this.addCount[index]++;
}
},
minusScore: function(index) {
if (this.minusCount[index] < 2) {
this.courses[index].score -= Math.floor(Math.random() * 10) + 1;
this.minusCount[index]++;
}
if (this.minusCount[index] >= 2 && this.minusCount[index] <= 4) {
this.courses[index].score -= 10;
}
},
calculateGPA: function(score) {
if (score >= 90) {
return 4.0;
} else if (score >= 85) {
return 3.7;
} else if (score >= 82) {
return 3.3;
} else if (score >= 78) {
return 3.0;
} else if (score >= 75) {
return 2.7;
} else if (score >= 72) {
return 2.3;
} else if (score >= 68) {
return 2.0;
} else if (score >= 64) {
return 1.5;
} else if (score >= 60) {
return 1.0;
} else {
return 0.0;
}
}
},
computed: {
totalScore: function() {
var sum = 0;
for (var i = 0; i < this.courses.length; i++) {
sum += this.courses[i].score;
}
return sum;
},
averageScore: function() {
return (this.totalScore / this.courses.length).toFixed(2);
},
totalGPA: function() {
var sum = 0;
for (var i = 0; i < this.courses.length; i++) {
sum += this.calculateGPA(this.courses[i].score) * this.courses[i].credit;
}
return sum.toFixed(2);
},
averageGPA: function() {
return (this.totalGPA / this.courses.length).toFixed(2);
}
},
watch: {
addCount: function(newCount) {
var sum = 0;
for (var i = 0; i < newCount.length; i++) {
sum += newCount[i];
}
if (sum >= 2 && sum <= 4) {
for (var i = 0; i < this.courses.length; i++) {
this.courses[i].score -= 10;
}
for (var i = 0; i < newCount.length; i++) {
newCount[i] = -1;
}
}
},
minusCount: function(newCount) {
var sum = 0;
for (var i = 0; i < newCount.length; i++) {
sum += newCount[i];
}
if (sum >= 2 && sum <= 4) {
for (var i = 0; i < this.courses.length; i++) {
this.courses[i].score -= 10;
}
for (var i = 0; i < newCount.length; i++) {
newCount[i] = -1;
}
}
}
}
})
</script>
</body>
</html>
相关推荐
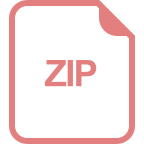
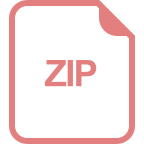
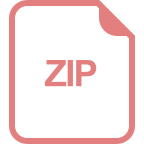














