给jtable设置单选框
时间: 2023-06-05 16:06:21 浏览: 74
好的,关于给JTable设置单选框的问题,你可以使用JRadioButton或JCheckBox作为单选框或复选框,然后结合TableCellRenderer和TableCellEditor对JTable的每个单元格进行个性化设置,以实现单选或多选的效果。详细实现方法可以参考相关的Java Swing教程或文档。
相关问题
给jtable设置单选框的代码和注释
以下是Java Swing中给JTable设置单选框的代码和注释:
```java
import javax.swing.*;
import javax.swing.table.DefaultTableModel;
import javax.swing.table.TableColumnModel;
import java.awt.*;
public class JTableRadioButton extends JFrame {
private JTable table;
public JTableRadioButton() {
setTitle("JTable Radio Button Example");
// 创建表数据
Object[][] data = {
{new Boolean(false), "John Doe", "1-555-555-5555"},
{new Boolean(false), "Mary Jane", "1-555-555-1234"},
{new Boolean(false), "Bob Smith", "1-555-555-9876"},
{new Boolean(false), "Jane Doe", "1-555-555-5555"}
};
// 创建表头
String[] columnNames = {"Selected", "Name", "Phone Number"};
// 创建表模型,并设置数据和表头
DefaultTableModel model = new DefaultTableModel(data, columnNames);
// 创建表并设置模型
table = new JTable(model);
// 获取表格控制器
TableColumnModel columnModel = table.getColumnModel();
// 获取单选框列,并将它的宽度设置为100像素
int radioButtonColumnIndex = 0;
columnModel.getColumn(radioButtonColumnIndex).setPreferredWidth(100);
// 设置单选框渲染器和编辑器
columnModel.getColumn(radioButtonColumnIndex).setCellRenderer(new RadioButtonRenderer());
columnModel.getColumn(radioButtonColumnIndex).setCellEditor(new RadioButtonEditor(new JCheckBox()));
// 将表格添加到窗口中
add(new JScrollPane(table));
// 设置窗口大小并可见
setSize(400, 200);
setVisible(true);
}
// 单选框渲染器
class RadioButtonRenderer implements TableCellRenderer {
private JRadioButton radioButton = new JRadioButton();
public Component getTableCellRendererComponent(JTable table, Object value, boolean isSelected, boolean hasFocus, int row, int column) {
if (value != null) {
radioButton.setSelected(((Boolean) value).booleanValue());
} else {
radioButton.setSelected(false);
}
return radioButton;
}
}
// 单选框编辑器
class RadioButtonEditor extends DefaultCellEditor implements ItemListener {
private JRadioButton radioButton;
public RadioButtonEditor(JCheckBox checkBox) {
super(checkBox);
radioButton = new JRadioButton();
radioButton.addItemListener(this);
}
public Component getTableCellEditorComponent(JTable table, Object value, boolean isSelected, int row, int column) {
if (value != null) {
radioButton.setSelected(((Boolean) value).booleanValue());
} else {
radioButton.setSelected(false);
}
return radioButton;
}
public Object getCellEditorValue() {
return Boolean.valueOf(radioButton.isSelected());
}
public void itemStateChanged(ItemEvent e) {
super.fireEditingStopped();
}
}
public static void main(String[] args) {
new JTableRadioButton();
}
}
```
注释中解释了代码中每个部分所做的事情。建议您看懂代码后再使用它,这样才能更好地理解它是如何实现的。
如何给jtable添加单选框
您好,可以使用JRadioButton作为单选框,然后将其添加到JTable的表格中,具体实现可以通过继承AbstractTableModel,重写getColumnClass方法,将对应列的Class设置为JRadioButton即可。具体代码实现如下:
```
import javax.swing.*;
import javax.swing.table.AbstractTableModel;
public class MyTableModel extends AbstractTableModel {
private Object[][] rowData = {
{"1", "option1"},
{"2", "option2"},
{"3", "option3"},
};
private String[] columnNames = {"ID", "Options"};
private JRadioButton[][] radioButtons = new JRadioButton[rowData.length][1];
@Override
public int getRowCount() {
return rowData.length;
}
@Override
public int getColumnCount() {
return columnNames.length;
}
@Override
public Object getValueAt(int rowIndex, int columnIndex) {
if (columnIndex == 0) {
return rowData[rowIndex][columnIndex];
} else {
JRadioButton radioButton = radioButtons[rowIndex][columnIndex - 1];
if (radioButton == null) {
radioButton = new JRadioButton();
radioButtons[rowIndex][columnIndex - 1] = radioButton;
}
return radioButton;
}
}
@Override
public String getColumnName(int column) {
return columnNames[column];
}
@Override
public Class<?> getColumnClass(int columnIndex) {
if (columnIndex == 0) {
return Integer.class;
} else {
return JRadioButton.class;
}
}
public static void main(String[] args) {
JFrame frame = new JFrame("JTable with JRadioButton");
frame.setSize(400, 300);
JTable table = new JTable(new MyTableModel());
JScrollPane scrollPane = new JScrollPane(table);
frame.add(scrollPane);
frame.setVisible(true);
frame.setLocationRelativeTo(null);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
```
阅读全文
相关推荐
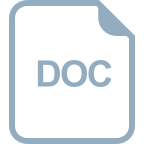
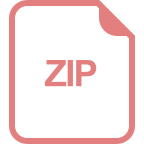







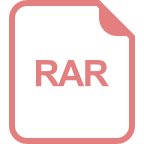
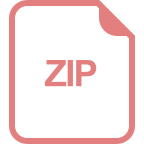
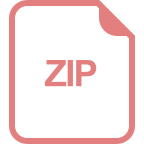