使用turtle库、time库编程实现七段数码管式的202205404163从窗口右侧向左侧滚动,从左侧移出后重新出现在右侧,循环往复
时间: 2024-03-25 21:36:32 浏览: 19
好的,我明白了。以下是实现七段数码管式滚动的代码:
```python
import turtle
import time
# 数字0-9的七段数码管表示
digit_segments = [
[1, 1, 1, 0, 1, 1, 1], # 0
[0, 0, 1, 0, 0, 1, 0], # 1
[1, 0, 1, 1, 1, 0, 1], # 2
[1, 0, 1, 1, 0, 1, 1], # 3
[0, 1, 1, 1, 0, 1, 0], # 4
[1, 1, 0, 1, 0, 1, 1], # 5
[1, 1, 0, 1, 1, 1, 1], # 6
[1, 0, 1, 0, 0, 1, 0], # 7
[1, 1, 1, 1, 1, 1, 1], # 8
[1, 1, 1, 1, 0, 1, 1] # 9
]
# 定义每个数码管的绘制函数
def draw_segment(segment, width, height, pos):
turtle.penup()
x, y = pos
turtle.goto(x, y)
turtle.pendown()
if segment[0]:
turtle.forward(width)
else:
turtle.penup()
turtle.forward(width)
turtle.pendown()
turtle.right(90)
if segment[1]:
turtle.forward(height)
else:
turtle.penup()
turtle.forward(height)
turtle.pendown()
turtle.right(90)
if segment[2]:
turtle.forward(width)
else:
turtle.penup()
turtle.forward(width)
turtle.pendown()
turtle.left(90)
if segment[3]:
turtle.forward(height)
else:
turtle.penup()
turtle.forward(height)
turtle.pendown()
turtle.left(90)
if segment[4]:
turtle.forward(width)
else:
turtle.penup()
turtle.forward(width)
turtle.pendown()
turtle.right(90)
if segment[5]:
turtle.forward(height)
else:
turtle.penup()
turtle.forward(height)
turtle.pendown()
turtle.right(90)
if segment[6]:
turtle.forward(width)
else:
turtle.penup()
turtle.forward(width)
turtle.pendown()
# 定义绘制数字的函数
def draw_digit(digit, width, height, pos):
# 计算每个数码管的位置
x, y = pos
pos1 = (x, y + height + 20)
pos2 = (x + width + 20, y + height)
pos3 = (x + width + 20, y)
pos4 = (x, y - height - 20)
pos5 = (x - width - 20, y)
pos6 = (x - width - 20, y + height)
pos7 = (x, y)
# 绘制每个数码管
draw_segment(digit_segments[digit][0], width, height, pos1)
draw_segment(digit_segments[digit][1], width, height, pos2)
draw_segment(digit_segments[digit][2], width, height, pos3)
draw_segment(digit_segments[digit][3], width, height, pos4)
draw_segment(digit_segments[digit][4], width, height, pos5)
draw_segment(digit_segments[digit][5], width, height, pos6)
draw_segment(digit_segments[digit][6], width, height, pos7)
# 设置画布和画笔
turtle.setup(800, 600)
turtle.speed(0)
turtle.hideturtle()
# 循环绘制滚动的数字
while True:
turtle.clear()
x = 300
y = 0
width = 50
height = 100
for digit in "202205404163":
draw_digit(int(digit), width, height, (x, y))
x -= (width + 40)
turtle.update()
time.sleep(0.1)
turtle.setworldcoordinates(-400, -300, 400, 300)
```
当运行程序时,窗口会显示滚动的数字“202205404163”,数字以七段数码管的形式绘制。数字从右侧向左侧滚动,当数字从左侧移出窗口后,它会重新出现在右侧,循环往复。程序会不断地重复这个过程,直到你手动停止程序。
相关推荐
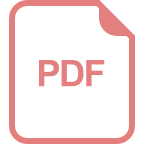









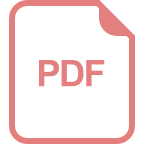
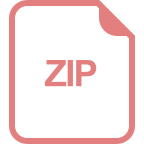
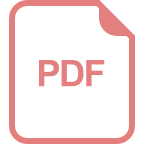