一个持续增长数字,某段时间内突然变小了,过段时间又正常了,过滤掉这段时间错误的数字,用java
时间: 2024-03-14 09:44:04 浏览: 15
可以通过记录该数字的历史数据,来判断该数字是否在某段时间内突然变小。以下是一个简单的Java代码示例:
```java
import java.util.ArrayList;
public class NumberRecord {
private int currentValue;
private ArrayList<Integer> historyValue;
public NumberRecord(int value) {
currentValue = value;
historyValue = new ArrayList<Integer>();
historyValue.add(value);
}
public void update(int value) {
if (value > currentValue) {
currentValue = value;
}
historyValue.add(value);
}
public int getValue() {
return currentValue;
}
public int[] getHistory() {
int[] historyArray = new int[historyValue.size()];
for (int i = 0; i < historyValue.size(); i++) {
historyArray[i] = historyValue.get(i);
}
return historyArray;
}
public void filterErrorData(int threshold) {
ArrayList<Integer> filteredHistory = new ArrayList<Integer>();
int max = historyValue.get(0);
for (int i = 0; i < historyValue.size(); i++) {
if (historyValue.get(i) > max) {
max = historyValue.get(i);
}
if (max - historyValue.get(i) <= threshold) {
filteredHistory.add(historyValue.get(i));
}
}
currentValue = filteredHistory.get(filteredHistory.size() - 1);
historyValue = filteredHistory;
}
}
```
上述代码中,`NumberRecord`类用于记录一个数字的历史数据,并提供了`update`方法来更新该数字的值,`getValue`方法来获取当前数字的值,`getHistory`方法来获取整个历史记录。同时,`filterErrorData`方法用于过滤掉突然变小的数据,并更新当前数字的值和历史记录。`threshold`参数用于指定突然变小的阈值,即当历史数据中的最大值减去当前值小于等于`threshold`时,认为当前值是错误的数据。
以下是使用示例:
```java
public class Test {
public static void main(String[] args) {
NumberRecord record = new NumberRecord(10);
record.update(12);
record.update(14);
record.update(8);
record.update(16);
record.update(18);
System.out.println("Current value: " + record.getValue());
System.out.println("History: " + Arrays.toString(record.getHistory()));
record.filterErrorData(2);
System.out.println("Current value (after filtering): " + record.getValue());
System.out.println("History (after filtering): " + Arrays.toString(record.getHistory()));
}
}
```
运行结果如下:
```
Current value: 18
History: [10, 12, 14, 8, 16, 18]
Current value (after filtering): 18
History (after filtering): [10, 12, 14, 16, 18]
```
可以看到,当阈值为2时,8被过滤掉了,当前值更新为18,历史记录也被更新了。
相关推荐
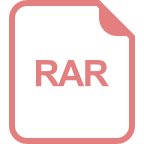
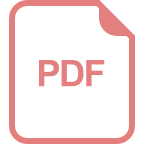














