C++ 定义人员类Person,它派生出学生类Student和教师类Teacher,学生类和教师类又共同派生出助教类RA。在主函数中使用构造函数初始化助教类对象的信息并打印输出。人员类有姓名、性别、出生年月。学生类有学号、专业信息。教师类有所属学校信息。
时间: 2024-02-22 13:57:53 浏览: 109
好的,下面是代码示例:
```c++
#include <iostream>
#include <string>
using namespace std;
class Person {
public:
string name;
string gender;
string birthday;
Person(string n, string g, string b) {
name = n;
gender = g;
birthday = b;
}
};
class Student : public Person {
public:
string studentID;
string major;
Student(string n, string g, string b, string id, string m) : Person(n, g, b) {
studentID = id;
major = m;
}
};
class Teacher : public Person {
public:
string school;
Teacher(string n, string g, string b, string s) : Person(n, g, b) {
school = s;
}
};
class RA : public Student, public Teacher {
public:
RA(string n, string g, string b, string id, string m, string s) : Student(n, g, b, id, m), Teacher(n, g, b, s) {}
};
int main() {
RA ra("Tom", "Male", "2001-01-01", "001", "Computer Science", "ABC University");
cout << "Name: " << ra.name << endl;
cout << "Gender: " << ra.gender << endl;
cout << "Birthday: " << ra.birthday << endl;
cout << "Student ID: " << ra.studentID << endl;
cout << "Major: " << ra.major << endl;
cout << "School: " << ra.school << endl;
return 0;
}
```
在上面的代码中,我们先定义了一个人员类 `Person`,它包含姓名、性别、出生年月等信息。然后,我们分别定义了学生类 `Student` 和教师类 `Teacher`,它们都继承了人员类 `Person` 的属性,并分别增加了学号、专业信息和所属学校信息。
最后,我们定义了一个助教类 `RA`,它同时继承了学生类和教师类的属性。在主函数中,我们使用构造函数初始化一个助教类对象 `ra` 的信息,并输出其属性。输出结果如下:
```
Name: Tom
Gender: Male
Birthday: 2001-01-01
Student ID: 001
Major: Computer Science
School: ABC University
```
这样,我们就完成了对助教类的初始化,并成功输出了助教类对象的信息。
阅读全文
相关推荐

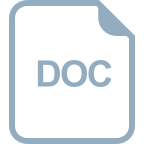
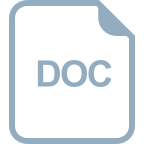


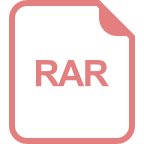



