void Set(char* p, const char* src) { p = new char[100]; strcpy_s(p, 100, src); } int main() { char* p = nullptr; Set(p, "hello world!"); cout << p << endl; delete p; return 0; }
时间: 2024-03-21 14:44:38 浏览: 52
这段代码存在一些问题。在函数`Set`中,参数`p`是一个指向指针的指针,因此我们可以通过修改指针`p`的值来改变指向的内存区域。但是在函数中,我们将`p`指向一个新的内存区域,这并不会影响到函数外部指针`p`所指向的内存区域。因此,程序输出的结果是不确定的,可能会导致未定义行为。此外,函数结束时,新分配的内存也没有被释放,可能会导致内存泄漏。应该改为以下代码:
```
void Set(char*& p, const char* src) {
if (p != nullptr) {
delete[] p;
}
p = new char[strlen(src)+1];
strcpy_s(p, strlen(src)+1, src);
}
int main() {
char* p = nullptr;
Set(p, "hello world!");
cout << p << endl;
delete[] p;
return 0;
}
```
这样修改后,函数`Set`中的参数`p`是一个指向指针的引用,通过修改引用来改变指针的值。同时在函数中,首先判断指针`p`是否已经指向了某个内存区域,如果已经指向,则需要先释放这块内存,然后再分配新的内存区域。在函数结束时,也要记得释放分配的内存。
相关问题
class Country { private: char name[40],capital[40]; int population; public: Country( const char* cou=NULL,const char* cap="NONE",int pop=0 ); const char* GetName const ( ); const char* GetCapital const ( ); int GetPopulation const ( ); void Set( const char* cou="Russia",const char* cap="Moscow",int pop=143000000 ); void Show(); }; Country::Country( const char* cou,const char* cap,int pop ) { strcpy(name,cou); strcpy(capital,cap); population=pop; } const char* Country::GetName const( ) { return name; } const char* Country::GetCapital const( ) { return capital; } int Country::GetPopulation const( ) { return population; } void Country::Set( const char* cou,const char* cap,int pop ) { strcpy(name,cou); strcpy(capital,cap); population=pop; } void Country::Show( ) { cout<<name<<"-"<<capital<<"-"<<population<<endl; } 有何问题
这段代码仍然有一个问题:
在定义成员函数时,函数名后的const关键字应该放在函数参数列表的后面,而不是放在函数名后面。
修正后的代码如下:
```
class Country {
private:
char name[40], capital[40];
int population;
public:
Country(const char* cou = NULL, const char* cap = "NONE", int pop = 0);
const char* GetName() const;
const char* GetCapital() const;
int GetPopulation() const;
void Set(const char* cou = "Russia", const char* cap = "Moscow", int pop = 143000000);
void Show();
};
Country::Country(const char* cou, const char* cap, int pop) {
strcpy(name, cou);
strcpy(capital, cap);
population = pop;
}
const char* Country::GetName() const {
return name;
}
const char* Country::GetCapital() const {
return capital;
}
int Country::GetPopulation() const {
return population;
}
void Country::Set(const char* cou, const char* cap, int pop) {
strcpy(name, cou);
strcpy(capital, cap);
population = pop;
}
void Country::Show() {
cout << name << "-" << capital << "-" << population << endl;
}
```
class Student { private: int Id, score; char name[9]; public: Student(int id = 10000, const char* n = NULL, int s = 0); int GetId() const; const char* GetName() const; int GetScore() const; void Set(int id = 99999, const char* n = "NONAME", int s = 100); void Show() const; }; Student::Student( int id,const char* n,int s ) { Id=id; score=s; strcpy(name,n); } int Student::GetId() const { return Id; } int Student::GetScore() const { return score; } const char* Student::GetName() const { return name; } void Student::Set( int id,const char* n,int s ) { Id=id; score=s; strcpy(name,n); } void Student::Show() const { cout<<Id<<"-"<<name<<"-"<<score<<endl;}这段代码有何问题
这段代码没有明显的语法错误,但是存在一些潜在问题:
1. char数组name[9]可能无法容纳超过8个字符的字符串,如果传入的n参数长度大于8,会出现问题。
2. 在构造函数中,如果传入的n为NULL指针,会导致strcpy函数出错。
3. 在Set函数中,如果传入的n为NULL指针,会导致strcpy函数出错。
4. 在Show函数中,没有考虑name中存在空格的情况,输出可能会出现问题。
5. 没有对传入的id和score进行有效范围的检查,可能会出现不合法的值。
阅读全文
相关推荐
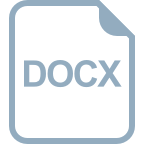
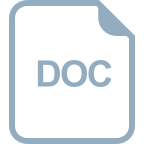
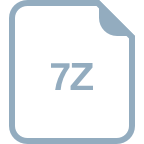






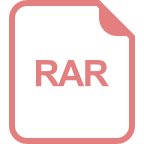
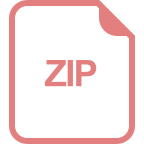
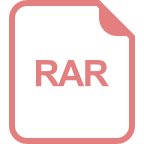