c++中的多线函数和linux中的多线程函数分别有哪些,全部给出函数原型
时间: 2023-12-04 16:05:37 浏览: 29
以下是C++标准库中常用的多线程函数的函数原型:
1. `std::thread`:
```cpp
namespace std {
class thread {
public:
thread() noexcept;
template< class Function, class... Args >
explicit thread( Function&& f, Args&&... args );
~thread();
thread( const thread& ) = delete;
thread& operator=( const thread& ) = delete;
thread( thread&& other ) noexcept;
thread& operator=( thread&& other ) noexcept;
void swap( thread& other ) noexcept;
bool joinable() const noexcept;
void join();
void detach();
id get_id() const noexcept;
static unsigned int hardware_concurrency() noexcept;
};
}
```
2. `std::mutex`:
```cpp
namespace std {
class mutex {
public:
mutex();
~mutex();
mutex( const mutex& ) = delete;
mutex& operator=( const mutex& ) = delete;
void lock();
bool try_lock();
void unlock();
};
}
```
3. `std::condition_variable`:
```cpp
namespace std {
class condition_variable {
public:
condition_variable();
~condition_variable();
condition_variable( const condition_variable& ) = delete;
condition_variable& operator=( const condition_variable& ) = delete;
void notify_one() noexcept;
void notify_all() noexcept;
void wait( unique_lock<mutex>& lock );
template< class Predicate >
void wait( unique_lock<mutex>& lock, Predicate pred );
template< class Clock, class Duration >
cv_status wait_until( unique_lock<mutex>& lock, const chrono::time_point<Clock,Duration>& abs_time );
template< class Clock, class Duration, class Predicate >
bool wait_until( unique_lock<mutex>& lock, const chrono::time_point<Clock,Duration>& abs_time, Predicate pred );
template< class Rep, class Period >
cv_status wait_for( unique_lock<mutex>& lock, const chrono::duration<Rep,Period>& rel_time );
template< class Rep, class Period, class Predicate >
bool wait_for( unique_lock<mutex>& lock, const chrono::duration<Rep,Period>& rel_time, Predicate pred );
};
}
```
4. `std::atomic`:
```cpp
namespace std {
template< class T >
struct atomic {
atomic() noexcept = default;
constexpr atomic( T desired ) noexcept;
atomic( const atomic& ) = delete;
atomic& operator=( const atomic& ) = delete;
T load( memory_order order = memory_order_seq_cst ) const noexcept;
void store( T desired, memory_order order = memory_order_seq_cst ) noexcept;
operator T() const noexcept;
T exchange( T desired, memory_order order = memory_order_seq_cst ) noexcept;
bool compare_exchange_weak( T& expected, T desired,
memory_order success = memory_order_seq_cst,
memory_order failure = memory_order_seq_cst ) noexcept;
bool compare_exchange_strong( T& expected, T desired,
memory_order success = memory_order_seq_cst,
memory_order failure = memory_order_seq_cst ) noexcept;
atomic& operator=( T desired ) noexcept;
T operator++( int ) noexcept;
T operator--( int ) noexcept;
T operator++() noexcept;
T operator--() noexcept;
T operator+=( T arg ) noexcept;
T operator-=( T arg ) noexcept;
T operator&=( T arg ) noexcept;
T operator|=( T arg ) noexcept;
T operator^=( T arg ) noexcept;
};
}
```
以下是Linux系统中常用的多线程函数的函数原型:
1. `pthread_create`:
```cpp
#include <pthread.h>
int pthread_create(pthread_t *thread, const pthread_attr_t *attr,
void *(*start_routine) (void *), void *arg);
```
2. `pthread_join`:
```cpp
#include <pthread.h>
int pthread_join(pthread_t thread, void **retval);
```
3. `pthread_mutex_lock`:
```cpp
#include <pthread.h>
int pthread_mutex_lock(pthread_mutex_t *mutex);
```
4. `pthread_mutex_unlock`:
```cpp
#include <pthread.h>
int pthread_mutex_unlock(pthread_mutex_t *mutex);
```
5. `pthread_cond_wait`:
```cpp
#include <pthread.h>
int pthread_cond_wait(pthread_cond_t *cond, pthread_mutex_t *mutex);
```
6. `pthread_cond_signal`:
```cpp
#include <pthread.h>
int pthread_cond_signal(pthread_cond_t *cond);
```
相关推荐
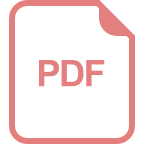
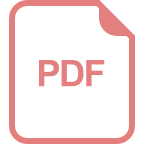
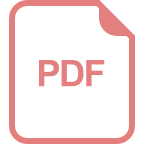














