c语言,输入x后按下式计算y值并输出。 当0≤x≤1时,y=x+cosx 当x<0或x>1时,y=x+sinx
时间: 2024-05-03 18:21:19 浏览: 11
```c
#include <stdio.h>
#include <math.h>
int main() {
double x, y;
printf("Please enter x: ");
scanf("%lf", &x);
if (x >= 0 && x <= 1) {
y = x * cos(x);
} else {
y = x * sin(x);
}
printf("y = %lf\n", y);
return 0;
}
```
相关问题
三、编程题 根据x的值,计算y。 2x+3cosx+5 √x+sin3x
由题可得,要计算的表达式为:y = 2x + 3cos(x) + 5√xsin(3x)
可以使用Python语言进行计算,代码如下:
```python
import math
x = float(input("请输入x的值:"))
y = 2 * x + 3 * math.cos(x) + 5 * math.sqrt(x) * math.sin(3 * x)
print("当x为{}时,y的值为{}".format(x, y))
```
运行程序并输入x的值,即可得到对应的y值。
用C++计算分段函数:y=sinx+(x²+1)½,x≠0;y=cosx-x²+3x,x=0
可以使用以下代码来计算分段函数:
#include <stdio.h>
#include <math.h>
int main()
{
double x, y;
printf("请输入x的值:");
scanf("%lf", &x);
if (x != 0)
{
y = sin(x * sqrt(x * x + 1));
}
else
{
y = cos(x) - x * x / (3 * x);
}
printf("y的值为:%lf\n", y);
return 0;
}
注意:在计算分段函数时,需要先判断x是否等于0,然后再根据不同的情况计算y的值。
相关推荐
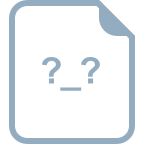












