在JavaScript中如何表达以下代码?var app = new PIXI.Application(600,500); document.body.appendChild(app.view); var bg = new PIXI.Sprite.fromImage("res/pao/ground01_2.png"); app.stage.addChild(bg); bg.y = 300; var ball = new PIXI.Sprite.fromImage("res/lianxi/llball/ball.png"); app.stage.addChild(ball); ball.y = 240; 1、小球向右移动(1像素/帧)。 2、在移动到坑的位置时,向下移动,实现(垂直)掉落坑中的动画效果。 3、小球掉落位置范围(280-440)
时间: 2023-06-14 08:06:05 浏览: 204
var app = new PIXI.Application(600, 500);
document.body.appendChild(app.view);
var bg = PIXI.Sprite.fromImage("res/pao/ground01_2.png");
bg.y = 300;
app.stage.addChild(bg);
var ball = PIXI.Sprite.fromImage("res/lianxi/llball/ball.png");
ball.y = 240;
app.stage.addChild(ball);
function animate() {
requestAnimationFrame(animate);
ball.x += 1;
if (ball.x >= 400 && ball.y < 440) {
ball.y += 1;
}
}
animate();
相关问题
在JavaScript中,var app = new PIXI.Application(500,600); document.body.appendChild(app.view); var bg = new PIXI.Sprite.fromImage("res/bg_01.png"); app.stage.addChild(bg); var bullet = new PIXI.Sprite.fromImage("res/plane/bullet/img_bullet_14.png"); bullet.anchor.set(0.5,0.5); app.stage.addChild(bullet); var plane = new PIXI.Sprite.fromImage("res/plane_blue_01.png"); plane.anchor.set(0.5,0.5); plane.x = 250; plane.y = 500; app.stage.addChild(plane); bg.interactive = true; bg.on("mousemove",movePlane); function movePlane(event){ var pos = event.data.getLocalPosition(app.stage); plane.x = pos.x; plane.y = pos.y; }, 添加帧频函数:animate,用于设置子弹 bullet 向上移动(30像素/帧) 当 bullet y < -50时,设置bullet y:plane.y-50、bullet x: plane.x。
可以在代码中添加如下的 animate 函数,用于设置子弹 bullet 向上移动并更新位置:
```
function animate() {
requestAnimationFrame(animate);
bullet.y -= 30;
if (bullet.y < -50) {
bullet.y = plane.y - 50;
bullet.x = plane.x;
}
app.renderer.render(app.stage);
}
```
然后在创建 PIXI 应用程序后调用 animate 函数即可:
```
var app = new PIXI.Application(500, 600);
document.body.appendChild(app.view);
// ...
animate();
```
在JavaScript中编写一个程序:1、创建 movespeed 函数(非带参函数),具体内容如下 (1)当小球 x 坐标移动到 922 时,设置 speed = -2;当小球 x 坐标移动到 86 时,设置 speed = 2;返回 speed 的值。 2、在 animate 帧频函数中控制小球的 x 轴移动,移动速度为 movespeed 函数的返回值/帧。以下是部分代码:var app = new PIXI.Application(1008,640); document.body.appendChild(app.view); var bj = new PIXI.Sprite.fromImage("res/lianxi/collision/bg.png"); app.stage.addChild(bj); var ball = new PIXI.Sprite.fromImage("res/lianxi/collision/qiu2.png"); ball.anchor.set(0.5,0.5); ball.x = 86; ball.y = 554; app.stage.addChild(ball) var speed = 2; //代码编写区域 app.ticker.add(animate); function animate(){ }
以下是在JavaScript中编写的程序:
```javascript
function movespeed() {
if (ball.x >= 922) {
speed = -2;
} else if (ball.x <= 86) {
speed = 2;
}
return speed;
}
var app = new PIXI.Application(1008, 640);
document.body.appendChild(app.view);
var bj = new PIXI.Sprite.fromImage("res/lianxi/collision/bg.png");
app.stage.addChild(bj);
var ball = new PIXI.Sprite.fromImage("res/lianxi/collision/qiu2.png");
ball.anchor.set(0.5, 0.5);
ball.x = 86;
ball.y = 554;
app.stage.addChild(ball);
var speed = 2;
app.ticker.add(animate);
function animate() {
ball.x += movespeed() / app.ticker.FPS;
}
```
在上述代码中,创建了一个名为 movespeed 的函数,用于控制小球的移动速度。在 animate 帧频函数中,通过调用 movespeed 函数来获取小球的移动速度,并将其除以当前的帧率,以确保小球每一帧移动的距离相同。最后,将计算出的移动距离加上小球的当前 x 坐标,以实现小球的移动。
阅读全文
相关推荐
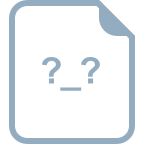
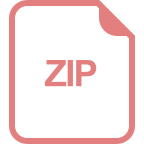
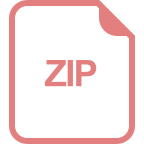



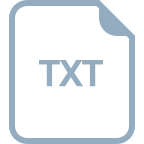
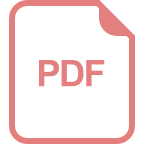
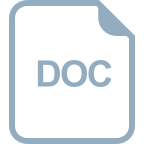

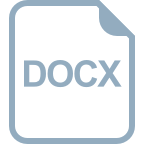
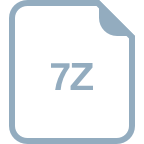
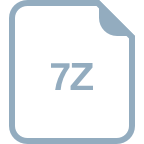
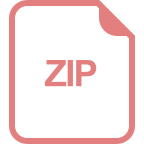