用c++创建表实现折半查找的完整代码
时间: 2024-05-15 14:12:47 浏览: 16
下面是一个使用 C++ 实现折半查找的完整代码,其中表的类型为数组:
```
#include <iostream>
using namespace std;
const int MAX_SIZE = 100;
struct Table {
int data[MAX_SIZE];
int length;
};
int binarySearch(Table table, int key) {
int low = 0;
int high = table.length - 1;
while (low <= high) {
int mid = (low + high) / 2;
if (table.data[mid] == key) {
return mid;
} else if (table.data[mid] < key) {
low = mid + 1;
} else {
high = mid - 1;
}
}
return -1;
}
int main() {
Table table = {{1, 3, 5, 7, 9, 11, 13, 15, 17, 19}, 10};
int key = 11;
int index = binarySearch(table, key);
if (index != -1) {
cout << "The index of the key " << key << " is " << index << endl;
} else {
cout << "The key " << key << " is not found." << endl;
}
return 0;
}
```
这个代码定义了一个 `Table` 结构体来表示表,包含一个 `data` 数组和 `length` 成员变量。`binarySearch` 函数实现了折半查找的算法,它接受一个 `Table` 类型的表和要查找的关键字 `key`,返回关键字在表中的索引,如果没有找到则返回 -1。在 `main` 函数中,我们定义了一个包含 10 个元素的表,并在其中查找关键字为 11 的元素。
相关推荐
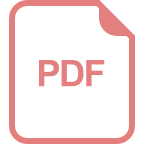
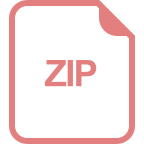
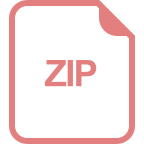
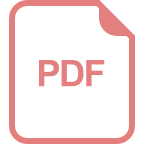
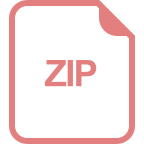
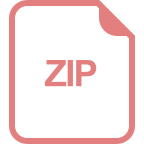
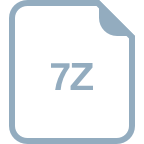
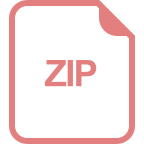