用c++写一个顺序结构的查找表,包括顺序查找和折半查找
时间: 2024-02-13 19:06:58 浏览: 106
以下是使用 C++ 实现一个顺序结构的查找表,包括顺序查找和折半查找的示例代码:
```
#include <iostream>
using namespace std;
#define MAX_SIZE 100 // 表的最大长度
typedef struct {
int key; // 关键字
// 其他数据项
} ElemType;
class SSTable {
public:
SSTable() { length = 0; } // 初始化表长为0
void insert(int k) { // 插入关键字为k的数据项
if (length >= MAX_SIZE) { // 表满,插入失败
cout << "Insert failed: table is full." << endl;
return;
}
elem[length].key = k;
length++;
}
int searchSeq(int k) { // 顺序查找关键字为k的数据项,返回其下标,没有则返回-1
for (int i = 0; i < length; i++) {
if (elem[i].key == k) {
return i;
}
}
return -1;
}
int searchBin(int k) { // 折半查找关键字为k的数据项,返回其下标,没有则返回-1
int low = 0, high = length - 1;
while (low <= high) {
int mid = (low + high) / 2;
if (elem[mid].key == k) {
return mid;
} else if (elem[mid].key < k) {
low = mid + 1;
} else {
high = mid - 1;
}
}
return -1;
}
void print() { // 打印表中元素
for (int i = 0; i < length; i++) {
cout << elem[i].key << " ";
}
cout << endl;
}
private:
ElemType elem[MAX_SIZE]; // 存储空间
int length; // 表长
};
int main() {
SSTable table;
table.insert(1);
table.insert(3);
table.insert(5);
table.insert(7);
table.print();
int index = table.searchSeq(5);
if (index == -1) {
cout << "Sequential search: Not found." << endl;
} else {
cout << "Sequential search: Found at index " << index << endl;
}
index = table.searchBin(5);
if (index == -1) {
cout << "Binary search: Not found." << endl;
} else {
cout << "Binary search: Found at index " << index << endl;
}
index = table.searchSeq(9);
if (index == -1) {
cout << "Sequential search: Not found." << endl;
} else {
cout << "Sequential search: Found at index " << index << endl;
}
index = table.searchBin(9);
if (index == -1) {
cout << "Binary search: Not found." << endl;
} else {
cout << "Binary search: Found at index " << index << endl;
}
return 0;
}
```
在这个示例代码中,我们在 `SSTable` 类中新增了 `searchSeq()` 和 `searchBin()` 方法,用于顺序查找和折半查找。在 `main()` 函数中,我们创建了一个 `SSTable` 对象,并向其中插入了一些数据项,然后进行了查找操作。
阅读全文
相关推荐

















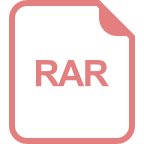