/** * 前端智能排序 */ @IgnoreAuth @RequestMapping("/autoSort") public R autoSort(@RequestParam Map<String, Object> params,JingdianxinxiEntity jingdianxinxi, HttpServletRequest request,String pre){ EntityWrapper<JingdianxinxiEntity> ew = new EntityWrapper<JingdianxinxiEntity>(); Map<String, Object> newMap = new HashMap<String, Object>(); Map<String, Object> param = new HashMap<String, Object>(); Iterator<Map.Entry<String, Object>> it = param.entrySet().iterator(); while (it.hasNext()) { Map.Entry<String, Object> entry = it.next(); String key = entry.getKey(); String newKey = entry.getKey(); if (pre.endsWith(".")) { newMap.put(pre + newKey, entry.getValue()); } else if (StringUtils.isEmpty(pre)) { newMap.put(newKey, entry.getValue()); } else { newMap.put(pre + "." + newKey, entry.getValue()); } } params.put("sort", "clicktime"); params.put("order", "desc"); PageUtils page = jingdianxinxiService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, jingdianxinxi), params), params)); return R.ok().put("data", page); }解释一下这个代码‘
时间: 2024-04-27 20:22:22 浏览: 35
这是一个后端接口的方法,用于实现前端智能排序功能。首先,它使用了 @IgnoreAuth 注解,表示不需要进行用户身份验证。然后,通过 @RequestMapping 注解设置了接口的访问路径为 "/autoSort"。接着,该方法接收三个参数:params、jingdianxinxi、request,以及一个名为 pre 的字符串参数。
在方法体中,首先创建了一个 EntityWrapper 对象 ew,用于构建 SQL 查询语句。然后,通过一个 while 循环,将 param 中的键值对存储到 newMap 中,新的键值对的键名是在原键名前加上 pre 后缀。接下来,将 "clicktime" 和 "desc" 分别设置为 params 中的 "sort" 和 "order" 的值,用于指定查询结果的排序方式。最后,调用 jingdianxinxiService 的 queryPage 方法查询符合条件的数据,并将结果以 JSON 格式返回给前端。
相关问题
@requestmapping /**
@RequestMapping注解是Spring MVC框架中的一个注解,用于将HTTP请求映射到控制器的处理方法上。
在引用和引用中,@RequestMapping注解被用于指定处理请求的URL路径和请求方法。例如,@RequestMapping(value="/editItem", method=RequestMethod.GET)表示当收到GET请求且路径为"/editItem"时,会调用相应的处理方法。
引用提供了一些使用@RequestMapping注解的示例。在这些示例中,@RequestMapping注解用于指定处理请求的URL路径和请求方法。例如,@RequestMapping(value="/hello", method=RequestMethod.GET)表示当收到GET请求且路径为"/hello"时,会调用hello()方法。
此外,@RequestMapping注解还可以用于指定多个URL路径和多个请求方法,以及其他参数,例如请求的媒体类型等。
总结起来,@RequestMapping注解是用于将HTTP请求映射到控制器的处理方法上的注解。它通过指定URL路径和请求方法来匹配请求,并调用相应的处理方法来处理请求。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* [@RequestMapping 用法详解](https://blog.csdn.net/weixin_40009737/article/details/80810918)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *3* [RequestMapping 使用教程](https://blog.csdn.net/xsj_blog/article/details/83150755)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
public R list(@RequestParam Map<String, Object> params,BaoxiuxinxiEntity baoxiuxinxi, HttpServletRequest request)
这是一个 Java 方法,方法名为 `list`,返回值类型为 `R`。该方法接收三个参数:
- `params`:类型为 `Map<String, Object>`,用于接收查询参数。
- `baoxiuxinxi`:类型为 `BaoxiuxinxiEntity`,表示一个维修信息实体类,用于接收查询参数。
- `request`:类型为 `HttpServletRequest`,表示一个 HTTP 请求对象。
该方法主要用于查询维修信息列表,并返回一个 `R` 类型的响应结果。其中,`@RequestParam` 注解用于将查询参数绑定到 `params` 参数上,`@RequestBody` 注解用于将 POST 请求体绑定到 `baoxiuxinxi` 参数上。
下面是一个示例代码:
```java
@RestController
@RequestMapping("/baoxiu")
public class BaoxiuController {
@Autowired
private BaoxiuService baoxiuService;
@GetMapping("/list")
public R list(@RequestParam Map<String, Object> params, BaoxiuxinxiEntity baoxiuxinxi, HttpServletRequest request) {
QueryWrapper<BaoxiuxinxiEntity> wrapper = new QueryWrapper<>(baoxiuxinxi);
wrapper.allEq(params);
PageUtils page = baoxiuService.queryPage(params, wrapper);
return R.ok().put("page", page);
}
// 其他方法省略...
}
```
在上面的示例代码中,使用 `@GetMapping` 注解标识处理 HTTP GET 请求的方法,请求路径为 `/baoxiu/list`。在方法中,首先创建一个 `QueryWrapper` 对象,将 `baoxiuxinxi` 参数传入其中,然后使用 `wrapper.allEq(params)` 方法将查询参数绑定到查询条件中。最后调用 `baoxiuService.queryPage` 方法查询维修信息列表,并将查询结果封装为一个 `PageUtils` 对象返回。
相关推荐
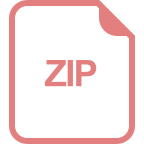
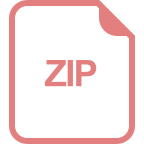
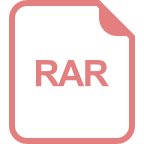











