js(1)单击“添加”按钮,将文本框中输入内容显示在下方的列表中。如果文本框内容为空,则弹出提示框“请输入待办事项”。(2)单击“删除”按钮,将当前列表项删除。 (3)单击“完成”按钮,将当前列表项的背景色设置为绿色。
时间: 2024-01-21 20:19:08 浏览: 21
以下是实现该功能的示例代码:
HTML:
```html
<!DOCTYPE html>
<html>
<head>
<title>Todo List</title>
<style type="text/css">
body {
margin: 0;
padding: 0;
background-color: #f6f6f6;
font-family: Arial, sans-serif;
font-size: 16px;
}
.container {
max-width: 600px;
margin: 50px auto;
background-color: #fff;
padding: 20px;
border-radius: 10px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.2);
}
.title {
margin-bottom: 20px;
font-size: 24px;
font-weight: bold;
text-align: center;
}
.input-box {
display: flex;
margin-bottom: 20px;
}
.input-box input[type="text"] {
flex: 1;
padding: 10px;
border-radius: 5px;
border: 1px solid #ccc;
font-size: 16px;
box-shadow: none;
outline: none;
}
.input-box button {
padding: 10px;
background-color: #008000;
color: #fff;
border-radius: 5px;
border: none;
cursor: pointer;
font-size: 16px;
margin-left: 10px;
box-shadow: none;
outline: none;
}
.list {
list-style: none;
padding: 0;
margin: 0;
}
.list li {
padding: 10px;
margin-bottom: 10px;
background-color: #fff;
border-radius: 5px;
box-shadow: 0 0 5px rgba(0, 0, 0, 0.1);
display: flex;
align-items: center;
justify-content: space-between;
}
.list li.completed {
background-color: #c1e1c1;
}
.list li button {
padding: 5px;
background-color: #ff0000;
color: #fff;
border-radius: 5px;
border: none;
cursor: pointer;
font-size: 16px;
margin-left: 10px;
box-shadow: none;
outline: none;
}
</style>
</head>
<body>
<div class="container">
<div class="title">Todo List</div>
<div class="input-box">
<input type="text" id="input" placeholder="请输入待办事项">
<button id="add-btn">添加</button>
</div>
<ul class="list" id="list">
</ul>
</div>
<script src="todo.js"></script>
</body>
</html>
```
JavaScript:
```javascript
// 获取元素
const input = document.getElementById('input');
const addBtn = document.getElementById('add-btn');
const list = document.getElementById('list');
// 添加按钮点击事件
addBtn.addEventListener('click', () => {
// 获取输入框内容
const value = input.value.trim();
// 判断是否为空
if (!value) {
alert('请输入待办事项');
return;
}
// 创建列表项
const li = document.createElement('li');
li.textContent = value;
// 创建删除按钮
const deleteBtn = document.createElement('button');
deleteBtn.textContent = '删除';
// 删除按钮点击事件
deleteBtn.addEventListener('click', () => {
li.remove();
});
// 创建完成按钮
const completeBtn = document.createElement('button');
completeBtn.textContent = '完成';
// 完成按钮点击事件
completeBtn.addEventListener('click', () => {
li.classList.add('completed');
});
// 将删除和完成按钮添加到列表项中
li.appendChild(deleteBtn);
li.appendChild(completeBtn);
// 将列表项添加到列表中
list.appendChild(li);
// 清空输入框内容
input.value = '';
});
```
相关推荐
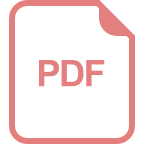
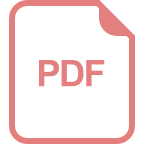















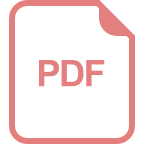