continue to use Java language, Add a Library class with the following UML specification: +-------------------------------------------+ | Library | +-------------------------------------------+ | - name: String | | - users: ArrayList<IUser> | +-------------------------------------------+ | + Library(String name) | | + addUser(IUser user): void | | + totalBorrowedBooks(): int | | + getBook(String name): int | | + moreBook(String name, int number): void | | + testLibrary(): void | +-------------------------------------------+ When a library is created, it has an arraylist of users (IUser) but the arraylist is empty (the arraylist does not contain any user). The addUser method takes a user (IUser) as argument and adds the user to the arraylist of users for the library. The totalBorrowedBooks method returns as result the total number of books borrowed by all users of the library (the result can be either positive or negative). The getBook method takes as argument the name of a user and returns as result the number of books currently borrowed by the user. If the library does not have a user with the given name, then the getBook method must throw an UnknownUserException with the message "User XXX unknown.", where XXX is replaced with the name of the user. Do not worry about multiple users having the same name. You can assume all user names are unique in the arraylist. The moreBook method takes as argument the name of a user and a number of books and changes the number of books currently borrowed by that user. If the library does not have a user with the given name, then the moreBook method must throw an UnknownUserException with the message "User XXX unknown.", where XXX is replaced with the name of the user. Do not worry about multiple users having the same name. Note: the moreBook method does not catch any exception, it only throws exceptions. Hint: use the equals method to compare strings, not the == operator which only works with constant strings.
时间: 2023-08-15 08:11:34 浏览: 49
Here is the Java code for the Library class:
```java
import java.util.ArrayList;
public class Library {
private String name;
private ArrayList<IUser> users;
public Library(String name) {
this.name = name;
this.users = new ArrayList<IUser>();
}
public void addUser(IUser user) {
this.users.add(user);
}
public int totalBorrowedBooks() {
int total = 0;
for (IUser user : this.users) {
total += user.getBorrowedBooks();
}
return total;
}
public int getBook(String name) throws UnknownUserException {
for (IUser user : this.users) {
if (user.getName().equals(name)) {
return user.getBorrowedBooks();
}
}
throw new UnknownUserException("User " + name + " unknown.");
}
public void moreBook(String name, int number) throws UnknownUserException {
for (IUser user : this.users) {
if (user.getName().equals(name)) {
user.setBorrowedBooks(user.getBorrowedBooks() + number);
return;
}
}
throw new UnknownUserException("User " + name + " unknown.");
}
public void testLibrary() {
IUser user1 = new User("Alice");
IUser user2 = new User("Bob");
IUser user3 = new User("Charlie");
this.addUser(user1);
this.addUser(user2);
this.addUser(user3);
try {
this.moreBook("Alice", 2);
this.moreBook("Bob", 3);
this.moreBook("Charlie", 4);
int total = this.totalBorrowedBooks();
System.out.println("Total borrowed books: " + total);
int aliceBooks = this.getBook("Alice");
System.out.println("Alice borrowed books: " + aliceBooks);
int bobBooks = this.getBook("Bob");
System.out.println("Bob borrowed books: " + bobBooks);
int charlieBooks = this.getBook("Charlie");
System.out.println("Charlie borrowed books: " + charlieBooks);
this.moreBook("Alice", -1);
aliceBooks = this.getBook("Alice");
System.out.println("Alice borrowed books: " + aliceBooks);
this.moreBook("Dave", 1);
} catch (UnknownUserException e) {
System.out.println(e.getMessage());
}
}
public static void main(String[] args) {
Library library = new Library("My Library");
library.testLibrary();
}
}
```
Here is the code for the IUser interface and the User class:
```java
public interface IUser {
public String getName();
public int getBorrowedBooks();
public void setBorrowedBooks(int borrowedBooks);
}
public class User implements IUser {
private String name;
private int borrowedBooks;
public User(String name) {
this.name = name;
this.borrowedBooks = 0;
}
public String getName() {
return this.name;
}
public int getBorrowedBooks() {
return this.borrowedBooks;
}
public void setBorrowedBooks(int borrowedBooks) {
this.borrowedBooks = borrowedBooks;
}
}
```
Note that the User class implements the IUser interface, which defines the methods required by the Library class. The testLibrary method in the Library class creates three users, adds them to the library, and tests the various methods of the library. The main method simply creates a Library object and calls its testLibrary method.
相关推荐
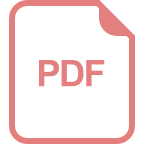
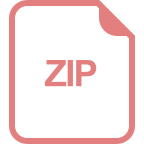














