帮我实现一个LFU缓存
时间: 2024-05-03 16:23:15 浏览: 19
这是一个 Python 实现的 LFU 缓存:
```python
from collections import defaultdict
class LFUCache:
def __init__(self, capacity):
self.capacity = capacity
self.cache = {}
self.freq = defaultdict(OrderedDict)
self.min_freq = 0
def get(self, key):
if key not in self.cache:
return -1
value, freq = self.cache[key]
self.freq[freq].pop(key)
if len(self.freq[freq]) == 0:
self.freq.pop(freq)
if self.min_freq == freq:
self.min_freq += 1
self.freq[freq+1][key] = value
self.cache[key] = (value, freq+1)
return value
def put(self, key, value):
if self.capacity == 0:
return
if key in self.cache:
self.cache[key] = (value, self.cache[key][1])
self.get(key)
return
if len(self.cache) == self.capacity:
k, v = self.freq[self.min_freq].popitem(last=False)
self.cache.pop(k)
self.cache[key] = (value, 1)
self.freq[1][key] = value
self.min_freq = 1
```
该实现中,LFU 缓存使用了一个字典 `cache` 来存储 key-value 对,使用一个字典 `freq` 来记录每个频次对应的 key-value 对,使用变量 `min_freq` 来记录当前最小的频次。
在 `get` 方法中,如果 key 不存在于 `cache` 中,则返回 -1。否则,将 key 对应的 value 和频次从 `freq` 中删除,如果该频次对应的 key-value 对已经为空,则将该频次从 `freq` 中删除,并更新 `min_freq`。然后,将 key 对应的 value 和频次加一后添加到 `freq` 中,并更新 `cache` 中的 key-value 对。
在 `put` 方法中,如果 `capacity` 为 0,则直接返回。如果 key 已存在于 `cache` 中,则将 `cache` 中的 key-value 对更新,并调用 `get` 方法以更新 `freq` 和 `min_freq`。如果 `cache` 已经满了,则从 `freq` 中删除当前最小频次的 key-value 对,并从 `cache` 中删除对应的 key-value 对。然后,将新的 key-value 对添加到 `cache` 和 `freq` 中,并将 `min_freq` 设置为 1。
使用示例:
```python
cache = LFUCache(2)
cache.put(1, 1)
cache.put(2, 2)
assert cache.get(1) == 1
cache.put(3, 3)
assert cache.get(2) == -1
assert cache.get(3) == 3
cache.put(4, 4)
assert cache.get(1) == -1
assert cache.get(3) == 3
assert cache.get(4) == 4
```
相关推荐
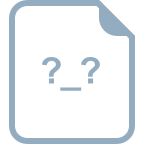
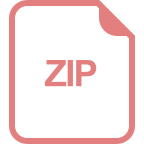
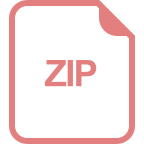














