解释代码static void led_update(void) { int j = 20; while(j--) { led_char_disp(); } }
时间: 2024-02-29 13:56:31 浏览: 51
这段代码定义了一个静态函数 `led_update()`,其返回类型为 `void`,表示不返回任何值。函数内部定义了一个整型变量 `j` 并初始化为 20。接下来进入一个 while 循环,当 j 不为零时执行循环体内的语句。
循环体内调用了一个函数 `led_char_disp()`,该函数用于控制 LED 灯的显示。循环执行 20 次,每次调用 `led_char_disp()` 函数,最终实现了 LED 灯的更新。
相关问题
lvgl利用定时器显示DS18B20温度数值代码
以下是一个基于lvgl的温度显示的示例代码,其中使用了定时器更新温度数值:
```c
#include "lvgl/lvgl.h"
#include "lvgl/lvgl_disp.h"
#include "lvgl/lvgl_theme.h"
#include "lvgl/lvgl.h"
#include "lv_drivers/display/fbdev.h"
#include "lv_drivers/indev/evdev.h"
#include <unistd.h>
#include <sys/ioctl.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#define TEMP_SENSOR_PATH "/sys/bus/w1/devices/28-00000xxxxxxx/w1_slave"
#define TEMP_SENSOR_ID "28-00000xxxxxxx"
static lv_obj_t *temp_label;
static void update_temp_value(lv_timer_t *timer)
{
char buf[128];
int fd = open(TEMP_SENSOR_PATH, O_RDONLY);
if (fd < 0) {
lv_label_set_text(temp_label, "Error");
return;
}
int len = read(fd, buf, sizeof(buf));
if (len < 0) {
lv_label_set_text(temp_label, "Error");
close(fd);
return;
}
buf[len] = '\0';
char *p = strstr(buf, "t=");
if (p == NULL) {
lv_label_set_text(temp_label, "Error");
close(fd);
return;
}
int temp = atoi(p+2);
temp = temp / 100.0;
snprintf(buf, sizeof(buf), "Temperature: %d C", temp);
lv_label_set_text(temp_label, buf);
close(fd);
}
int main(int argc, char *argv[])
{
/* Initialize LVGL */
lv_init();
/* Initialize frame buffer device driver */
fbdev_init();
/* Initialize input device driver */
evdev_init();
/* Create a screen */
lv_obj_t *screen = lv_disp_create_screen(NULL, NULL);
/* Create a style */
static lv_style_t style;
lv_style_init(&style);
lv_style_set_text_font(&style, LV_STATE_DEFAULT, &lv_font_roboto_28);
lv_style_set_text_color(&style, LV_STATE_DEFAULT, LV_COLOR_BLACK);
/* Create a label */
temp_label = lv_label_create(screen, NULL);
lv_label_set_text(temp_label, "Loading...");
lv_obj_add_style(temp_label, LV_LABEL_PART_MAIN, &style);
lv_obj_align(temp_label, NULL, LV_ALIGN_CENTER, 0, 0);
/* Create a timer to update temperature value */
lv_timer_create(update_temp_value, 1000, NULL);
/* Start the main loop */
while (1) {
lv_task_handler();
usleep(1000);
}
return 0;
}
```
注意,此代码假设你已经连接了 DS18B20 温度传感器并且已经将其连接到了正确的 GPIO 引脚上。在这个示例中,我们从 `/sys/bus/w1/devices/28-00000xxxxxxx/w1_slave` 文件中读取温度值,其中 `28-00000xxxxxxx` 是你的传感器的 ID,你需要将其替换为你自己传感器的 ID。此外,我们使用定时器每秒钟更新一次温度值,你可以根据自己的需要调整此时间间隔。
使用arduino+esp32+tft_espi+lvgl+心知天气写一个网路天气时钟的代码
### 回答1:
很高兴为您提供帮助! 下面是一个简单的示例代码,您需要将其修改为适合您的硬件和网络设置的版本。
```
#include <WiFi.h>
#include <WiFiClientSecure.h>
#include <HTTPClient.h>
#include <ArduinoJson.h>
#include <TFT_eSPI.h>
#include <lvgl.h>
// 修改为您的 WiFi SSID 和密码
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
// 修改为您在心知天气注册的 API Key 和城市 ID
const char* apiKey = "your_API_KEY";
const char* cityId = "your_CITY_ID";
// 修改为您的 TFT_eSPI 硬件设置
TFT_eSPI tft = TFT_eSPI();
#define TFT_WIDTH 240
#define TFT_HEIGHT 240
void setup() {
Serial.begin(115200);
// 初始化 TFT_eSPI 硬件
tft.begin();
tft.setRotation(1);
tft.fillScreen(TFT_BLACK);
// 连接 WiFi
Serial.printf("Connecting to WiFi %s...\n", ssid);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.print(".");
}
Serial.println("");
Serial.printf("WiFi connected, IP address: %s\n", WiFi.localIP().toString().c_str());
// 初始化 LVGL
lv_init();
lv_disp_drv_t disp_drv;
lv_disp_drv_init(&disp_drv);
disp_drv.hor_res = TFT_WIDTH;
disp_drv.ver_res = TFT_HEIGHT;
disp_drv.flush_cb = [](lv_disp_drv_t* disp_drv, const lv_area_t* area, lv_color_t* color_p) {
tft.startWrite();
tft.setAddrWindow(area->x1, area->y1, area->x2, area->y2);
uint32_t size = (area->x2 - area->x1 + 1) * (area->y2 - area->y1 + 1);
tft.pushColors((uint16_t*)color_p, size, true);
tft.endWrite();
lv_disp_flush_ready(disp_drv);
};
lv_disp_drv_register(&disp_drv);
lv_theme_t* theme = lv_theme_material_init(210, NULL);
lv_theme_set_current(theme);
// 创建 LVGL 控件
lv_obj_t* label_time = lv_label_create(lv_scr_act(), NULL);
lv_obj_align(label_time, NULL, LV_ALIGN_CENTER, 0, -50);
lv_label_set_text(label_time, "Loading...");
lv_obj_t* label_date = lv_label_create(lv_scr_act(), NULL);
lv_obj_align(label_date, NULL, LV_ALIGN_CENTER, 0, 50);
lv_label_set_text(label_date, "Loading...");
// 更新天气和时间
updateWeather();
updateTime();
}
void loop() {
// 每分钟更新一次时间和天气
static uint32_t lastUpdateTime = 0;
if (millis() - lastUpdateTime >= 60000) {
updateWeather();
updateTime();
lastUpdateTime = millis();
}
// 处理 LVGL 事件
lv_task_handler();
delay(5);
}
void updateWeather() {
// 发送 HTTP 请求获取天气数据
WiFiClientSecure client;
if (!client.connect("api.seniverse.com", 443)) {
Serial.println("Failed to connect to weather server");
return;
}
String url = "/v3/weather/now.json?key=" + String(apiKey) + "&location=" + String(cityId);
client.print(String("GET ") + url + " HTTP/1.1\r\n" +
"Host: api.seniverse.com\r\n" +
"User-Agent: ESP32\r\n" +
"Connection: close\r\n\r\n");
unsigned long timeout = millis();
while (client.available() == 0) {
if (millis() - timeout > 5000) {
Serial.println("Failed to receive weather data");
return;
}
delay(100);
}
// 解析 JSON 数据
String line = client.readStringUntil('\n');
while (!line.startsWith("{")) {
line = client.readStringUntil('\n');
}
DynamicJsonDocument doc(1024);
DeserializationError error = deserializeJson(doc, line);
if (error) {
Serial.println("Failed to parse weather data");
return;
}
JsonObject weather = doc["results"][0]["now"];
const char* text = weather["text"];
int temperature = weather["temperature"];
// 更新 LVGL 控件
lv_obj_t* label_weather = lv_label_create(lv_scr_act(), NULL);
lv_obj_align(label_weather, NULL, LV_ALIGN_CENTER, 0, 100);
lv_label_set_text_fmt(label_weather, "%s %d°C", text, temperature);
}
void updateTime() {
// 获取当前时间
time_t now = time(nullptr);
struct tm* timeinfo = localtime(&now);
// 更新 LVGL 控件
lv_obj_t* label_time = lv_scr_act()->child_ll;
lv_label_set_text_fmt(label_time, "%02d:%02d", timeinfo->tm_hour, timeinfo->tm_min);
lv_obj_t* label_date = label_time->sibling_ll;
lv_label_set_text_fmt(label_date, "%04d-%02d-%02d", timeinfo->tm_year + 1900, timeinfo->tm_mon + 1, timeinfo->tm_mday);
}
```
请注意,此示例代码需要您在心知天气注册并获取 API Key 和城市 ID。此外,您需要安装 TFT_eSPI 和 LVGL 库,并将其与您的硬件设置匹配。
### 回答2:
使用Arduino ESP32 TFT_ESPI LVGL编写一个网络天气时钟的代码,可以实现以下功能:
1. 首先,我们需要连接ESP32开发板和TFT显示屏,确保它们可以正常通信。
2. 然后,我们需要设置心知天气的API密钥和要查询的城市。
3. 通过与心知天气API通信,获取当前天气信息,例如当前温度、天气状况等。
4. 将获取到的天气信息显示在TFT显示屏上,可以使用LVGL库提供的函数在屏幕上绘制不同的元素,如文字、图标等。
5. 设置一个时钟功能,实时更新天气信息。可以使用ESP32的定时器功能,在固定的时间间隔内执行获取天气数据和更新显示屏的操作。
6. 可以添加其他功能,如显示日期、闹钟功能等。
下面是一个简单的示例代码:
```cpp
#include <Arduino.h>
#include <TFT_eSPI.h>
#include <lvgl.h>
TFT_eSPI tft;
const char* apiKey = "YOUR_API_KEY";
const char* city = "YOUR_CITY";
void setup() {
tft.begin();
tft.setRotation(1);
lv_init();
lv_disp_drv_t disp_drv;
lv_disp_drv_init(&disp_drv);
disp_drv.disp_flush = tft.flush;
disp_drv.disp_fill = tft.fillScreen;
lv_disp_drv_register(&disp_drv);
}
lv_obj_t* label;
void updateWeather() {
// 使用HTTP请求从心知天气API获取当前天气数据,并更新显示屏上的天气信息
}
void updateClock() {
// 更新时钟显示
}
void loop() {
updateWeather();
updateClock();
delay(1000); // 每秒更新一次
}
```
以上代码是一个简化的示例,具体实现需要根据所使用的具体库和API进行适当的调整。可以根据LVGL库的文档,使用其提供的函数绘制各种元素,以实现更丰富的显示效果。同时,可以根据需要在代码中添加其他功能,以实现更多的定制化。
### 回答3:
编写一个网络天气时钟的代码需要以下步骤:
1. 配置Arduino IDE和ESP32开发环境:首先,确保你已经安装了Arduino IDE,并添加了ESP32开发板支持库。然后,将ESP32连接到电脑并选择正确的开发板和端口。
2. 安装必要的库:在Arduino IDE中,打开"工具"->"管理库",搜索并安装TFT_eSPI、lvgl以及心知天气的库。
3. 连接TFT显示屏:根据TFT显示屏的引脚定义,连接ESP32和TFT显示屏。通常,液晶显示屏的SCK、MISO、MOSI、CS、DC、RST和BL引脚需要与相应的ESP32引脚连接。
4. 获取心知天气的API密钥:在心知天气官网上注册账号并获取API密钥。这个密钥用于从心知天气获取实时天气数据。
5. 编写Arduino代码:使用Arduino IDE创建一个新的Sketch。导入必要的库,并根据TFT显示屏的引脚定义配置液晶显示屏。然后,在setup()函数中初始化TFT显示屏,并设置TFT的背景颜色和字体。
接下来,在loop()函数中,首先使用心知天气的API密钥,使用HTTP请求从心知天气获取实时天气数据。使用JSON解析器解析返回的JSON数据,并提取所需的天气信息,比如温度、天气状况等。
最后,使用TFT库提供的函数将所需的天气信息显示在TFT显示屏上。你可以选择显示当前时间、日期、温度和天气状况等等。
6. 上传代码:将完成的代码上传到ESP32开发板上,确保上传过程中没有错误。
7. 调试:如果你发现显示屏上没有显示预期的天气信息,可能需要检查你的代码逻辑和液晶显示屏的连接,以及心知天气API的使用是否正确。较大的问题可能需要进一步的调试和排查。
这个代码的实现需要一定的编程基础和对Arduino、ESP32、TFT_eSPI、lvgl和心知天气API的了解,但按照上述步骤和一些基本的编程知识,你应该能够顺利完成一个网络天气时钟的代码。
阅读全文
相关推荐
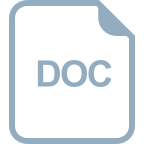
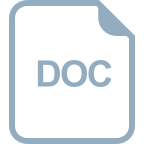
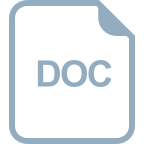
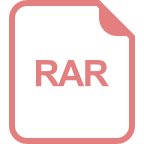
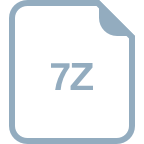
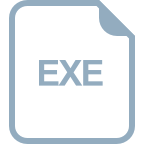





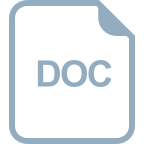
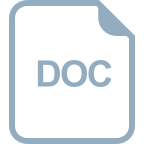
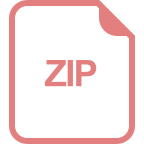
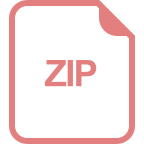
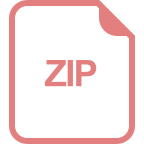