用c++实现模拟退火算法
时间: 2023-09-02 20:09:02 浏览: 55
以下是C++实现模拟退火算法的示例代码:
```cpp
#include <iostream>
#include <cmath>
#include <ctime>
using namespace std;
// 目标函数
double func(double x, double y) {
return sin(x) + cos(y);
}
int main() {
// 随机数种子
srand(time(nullptr));
// 初始温度和结束温度
double T = 100, T_end = 1e-8;
// 降温速率
double cooling_rate = 0.99;
// 初始状态
double x = rand() % 100, y = rand() % 100;
// 最优解
double best_x = x, best_y = y, best_val = func(x, y);
while (T > T_end) {
// 随机生成新状态
double new_x = x + (rand() % 21 - 10) * T;
double new_y = y + (rand() % 21 - 10) * T;
// 求解函数值
double val = func(new_x, new_y);
// 计算接受概率
double p = exp(-(val - best_val) / T);
// 判断是否接受新状态
if (p > static_cast<double>(rand()) / RAND_MAX) {
x = new_x;
y = new_y;
if (val > best_val) {
best_x = new_x;
best_y = new_y;
best_val = val;
}
}
// 降温
T *= cooling_rate;
}
cout << "Best solution: x = " << best_x << ", y = " << best_y << ", val = " << best_val << endl;
return 0;
}
```
注释已经很详细了,大概的思路就是从一个随机状态开始,每次随机生成新状态,计算接受概率,如果满足条件则接受新状态,否则继续在旧状态下搜索,同时不断降温,直到温度降至结束温度为止。最终输出找到的最优解。
相关推荐
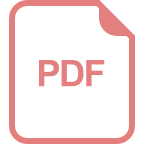














