学生信息包括:学号,姓名,年龄,性别,出生年月,地址,电话,E-mail等。试设计一学生信息管理系统,使之能提供以下功能: ●系统以菜单方式工作 ●学生信息录入功能(学生信息用文件保存)---输入 ●学生信息浏览功能---输出 ●查询、排序功能---算法 1、按学号查询 2、按姓名查询 ●学生信息的删除与修改(可选项) ●要求能通过文件保存信息,程序支持信息导入和保存 (2)功能要求 ●界面比较美观; ●有一定的容错能力,比如输入的成绩不在0~100之间,就提示不合法,要求重新输入; ●最好用链表的方式实现。
时间: 2024-02-25 19:57:08 浏览: 61
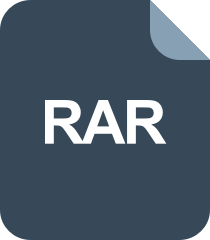
学生信息管理系统,实现学生信息包括:学号、姓名、性别、年龄、班级等信息。

好的,我可以为您设计一个学生信息管理系统,满足您的需求。以下是系统的基本功能:
1. 学生信息录入功能:用户可以输入学生信息,包括学号、姓名、年龄、性别、出生年月、地址、电话、E-mail等,系统将信息保存到文件中。
2. 学生信息浏览功能:用户可以查看已经录入的学生信息,包括学号、姓名、年龄、性别、出生年月、地址、电话、E-mail等。
3. 查询、排序功能:用户可以按学号或姓名查询学生信息,并可以按照学号或姓名对学生信息进行排序。
4. 学生信息的删除与修改:用户可以选择删除或修改已经录入的学生信息。
5. 信息导入和保存功能:用户可以将已经录入的学生信息保存到文件中,也可以从文件中导入学生信息。
以下是一个简单的实现,使用链表来存储学生信息:
```python
class Student:
def __init__(self, id, name, age, gender, birthday, address, phone, email):
self.id = id
self.name = name
self.age = age
self.gender = gender
self.birthday = birthday
self.address = address
self.phone = phone
self.email = email
self.next = None
class StudentList:
def __init__(self):
self.head = None
def add(self, student):
if self.head is None:
self.head = student
else:
current = self.head
while current.next is not None:
current = current.next
current.next = student
def find_by_id(self, id):
current = self.head
while current is not None:
if current.id == id:
return current
current = current.next
return None
def find_by_name(self, name):
current = self.head
while current is not None:
if current.name == name:
return current
current = current.next
return None
def remove(self, student):
if self.head == student:
self.head = student.next
else:
current = self.head
while current.next is not None:
if current.next == student:
current.next = student.next
break
current = current.next
def display(self):
current = self.head
while current is not None:
print(current.id, current.name, current.age, current.gender, current.birthday, current.address, current.phone, current.email)
current = current.next
def save_to_file(self, path):
with open(path, 'w') as f:
current = self.head
while current is not None:
f.write(f"{current.id},{current.name},{current.age},{current.gender},{current.birthday},{current.address},{current.phone},{current.email}\n")
current = current.next
def load_from_file(self, path):
self.head = None
with open(path, 'r') as f:
lines = f.readlines()
for line in lines:
fields = line.strip().split(',')
student = Student(fields[0], fields[1], fields[2], fields[3], fields[4], fields[5], fields[6], fields[7])
self.add(student)
```
这里,我们定义了两个类:`Student`类和`StudentList`类。`Student`类表示一个学生对象,包括学号、姓名、年龄、性别、出生年月、地址、电话、E-mail等属性。`StudentList`类表示学生信息的链表,可以对学生信息进行添加、删除、查找等操作。
该系统的基本UI界面可以使用Python的`tkinter`库来实现,但由于篇幅所限,这里就不再赘述。
阅读全文
相关推荐
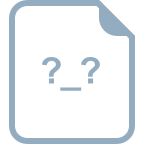
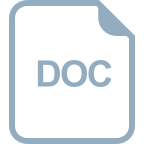













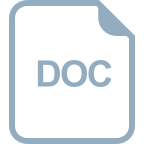
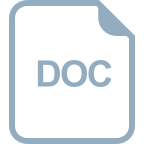