用c语言从键盘上输入n个数,要求用简单选择排序算法对这n个数按由大到小的顺序排序并输出。
时间: 2024-02-03 13:13:16 浏览: 29
以下是使用简单选择排序算法对输入的n个数按由大到小的顺序排序并输出的C语言代码:
```c
#include <stdio.h>
void selectionSort(int arr[], int n) {
int i, j, maxIndex;
for (i = 0; i < n-1; i++) {
maxIndex = i;
for (j = i+1; j < n; j++) {
if (arr[j] > arr[maxIndex]) {
maxIndex = j;
}
}
int temp = arr[i];
arr[i] = arr[maxIndex];
arr[maxIndex] = temp;
}
}
int main() {
int n, i;
printf("Enter the number of elements: ");
scanf("%d", &n);
int arr[n];
printf("Enter %d elements:\n", n);
for (i = 0; i < n; i++) {
scanf("%d", &arr[i]);
}
selectionSort(arr, n);
printf("Sorted array in descending order: ");
for (i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
```
首先,我们定义了一个 `selectionSort` 函数来实现简单选择排序算法。该函数接受一个整型数组 `arr` 和一个整数 `n` 作为参数。在函数中,我们使用两个嵌套的循环来遍历数组,并在每次内循环中找到当前未排序部分中的最大元素,并将其与未排序部分的第一个元素交换。这样,未排序部分中的最大元素就被移到了已排序部分的末尾。外循环继续遍历未排序部分,直到整个数组都被排序。
在 `main` 函数中,我们首先从用户输入中获取数组的大小 `n` 和数组的元素。然后,我们调用 `selectionSort` 函数来对数组进行排序,并输出结果。
相关推荐
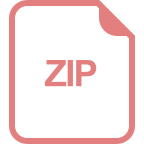














