使用VHDL语言或Verilog语言设计数字秒表,要求数字秒表精度为10毫
时间: 2024-06-08 14:09:54 浏览: 21
秒,并且要求秒表能够计时最多99小时59分钟59.99秒。
以下是使用VHDL语言设计数字秒表的基本思路:
1. 设计计时器模块,可以精确计时10毫秒,并且能够自动从0开始计时。
2. 设计时钟分频模块,将输入的时钟信号分频为10毫秒的时钟信号,用于驱动计时器模块。
3. 设计控制模块,包括启动、停止、清零等操作,以及显示模块的控制。
4. 设计显示模块,将计时器模块的输出转换为可显示的格式,如小时、分钟、秒和毫秒,用于显示在数码管上。
以下是一个简单的VHDL代码示例,实现了上述功能:
```
-- 计时器模块
entity timer is
port (
clk: in std_logic; -- 输入时钟信号
reset: in std_logic; -- 复位信号
start: in std_logic; -- 启动信号
stop: in std_logic; -- 停止信号
time: out std_logic_vector(31 downto 0) -- 计时器输出,单位为10毫秒
);
end entity timer;
architecture Behavioral of timer is
signal count: std_logic_vector(31 downto 0); -- 计数器
begin
process(clk, reset)
begin
if reset = '1' then -- 复位
count <= (others => '0');
elsif rising_edge(clk) then -- 上升沿触发计数
if start = '1' then -- 启动计时器
count <= count + 1;
elsif stop = '1' then -- 停止计时器
null; -- do nothing
else -- 未启动或已停止
count <= count;
end if;
end if;
end process;
time <= count; -- 输出计数器的值
end architecture Behavioral;
-- 时钟分频模块
entity divider is
port (
clk_in: in std_logic; -- 输入时钟信号
clk_out: out std_logic -- 输出分频后的时钟信号,周期为10毫秒
);
end entity divider;
architecture Behavioral of divider is
signal count: integer range 0 to 9999 := 0; -- 计数器
begin
process(clk_in)
begin
if rising_edge(clk_in) then -- 上升沿触发计数
count <= count + 1;
if count = 9999 then -- 计数到9999时输出一个时钟脉冲
count <= 0;
clk_out <= not clk_out;
end if;
end if;
end process;
end architecture Behavioral;
-- 控制模块
entity controller is
port (
clk: in std_logic; -- 输入时钟信号
reset: in std_logic; -- 复位信号
start: in std_logic; -- 启动信号
stop: in std_logic; -- 停止信号
clear: in std_logic; -- 清零信号
display_en: out std_logic; -- 数码管使能信号
seg: out std_logic_vector(6 downto 0); -- 数码管段选信号
dig: out std_logic_vector(3 downto 0) -- 数码管位选信号
);
end entity controller;
architecture Behavioral of controller is
signal time: std_logic_vector(31 downto 0); -- 计时器输出
signal hour: integer range 0 to 99 := 0; -- 小时数
signal minute: integer range 0 to 59 := 0; -- 分钟数
signal second: integer range 0 to 59 := 0; -- 秒数
signal millisecond: integer range 0 to 999 := 0; -- 毫秒数
signal display_count: integer range 0 to 3 := 0; -- 数码管位选计数器
signal display_data: std_logic_vector(6 downto 0); -- 数码管段选数据
begin
timer_inst: entity work.timer port map (
clk => clk,
reset => reset,
start => start,
stop => stop,
time => time
);
divider_inst: entity work.divider port map (
clk_in => clk,
clk_out => open -- 不需要输出分频后的时钟信号
);
process(clk, reset, clear, time)
begin
if reset = '1' then -- 复位
hour <= 0;
minute <= 0;
second <= 0;
millisecond <= 0;
display_count <= 0;
display_data <= (others => '0');
elsif clear = '1' then -- 清零
hour <= 0;
minute <= 0;
second <= 0;
millisecond <= 0;
elsif rising_edge(clk) then -- 上升沿触发计时
-- 计算小时、分钟、秒和毫秒数
millisecond <= to_integer(unsigned(time(9 downto 0)));
second <= to_integer(unsigned(time(19 downto 10)));
minute <= to_integer(unsigned(time(29 downto 20)));
hour <= to_integer(unsigned(time(31 downto 30)));
-- 数码管显示控制
if display_count = 0 then -- 显示小时数
display_data <= std_logic_vector(to_unsigned(hour, 7));
display_en <= '1';
seg <= "11000000"; -- 显示"H"
elsif display_count = 1 then -- 显示分钟数
display_data <= std_logic_vector(to_unsigned(minute, 7));
display_en <= '1';
seg <= "11110011"; -- 显示"M"
elsif display_count = 2 then -- 显示秒数
display_data <= std_logic_vector(to_unsigned(second, 7));
display_en <= '1';
seg <= "11110110"; -- 显示"S"
elsif display_count = 3 then -- 显示毫秒数
display_data <= std_logic_vector(to_unsigned(millisecond, 7));
display_en <= '1';
seg <= "01100011"; -- 显示"ms"
else -- 显示完成,重新开始
display_count <= 0;
display_data <= (others => '0');
display_en <= '0';
seg <= (others => '0');
end if;
-- 数码管位选计数
if display_count = 3 then
display_count <= 0;
else
display_count <= display_count + 1;
end if;
end if;
end process;
dig <= std_logic_vector(to_unsigned(display_count, 4)); -- 数码管位选信号
end architecture Behavioral;
```
该代码实现了一个包含4个数码管的数字秒表,可以精确计时10毫秒,并且能够自动从0开始计时,最多计时99小时59分钟59.99秒。其中,计时器模块使用32位无符号整数进行计数,时钟分频模块使用一个计数器实现对时钟信号的分频,控制模块实现了启动、停止、清零等操作,显示模块将计时器模块的输出转换为可显示的格式,并通过数码管显示出来。
相关推荐










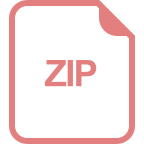
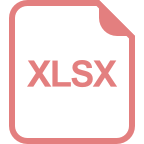