vue 子组件再watch中调用方法
时间: 2023-10-14 16:29:22 浏览: 89
可以在子组件的watch中调用父组件中的方法,方式如下:
在子组件中定义watch,监听需要的变量,如下:
```
watch: {
inputValue(val) {
this.$emit('input-change', val) // 调用父组件方法
}
}
```
在父组件模板中指定子组件,同时绑定方法,如下:
```
<template>
<child-component @input-change="handleInputChange" />
</template>
<script>
import ChildComponent from './ChildComponent'
export default {
components: { ChildComponent },
methods: {
handleInputChange(val) {
console.log(val) // 输出子组件中的inputValue值
}
}
}
</script>
```
这样,当子组件中的inputValue变化时,就会触发父组件中的handleInputChange方法。
相关问题
vue3 在watch中调用方法
在Vue3中,我们可以使用`watch`来监听数据的变化,并在数据变化时执行相应的操作。如果需要在`watch`中调用方法,可以将方法定义在`methods`中,然后在`watch`中使用箭头函数来调用该方法。具体步骤如下:
1. 在`setup`函数中定义`data`和`methods`:
```javascript
import { reactive } from 'vue'
export default {
setup() {
const state = reactive({
count: 0
})
const increment = () => {
state.count++
}
return {
state,
increment
}
}
}
```
2. 在`watch`中使用箭头函数调用`increment`方法:
```javascript
watch(() => state.count, () => {
console.log('count changed')
increment()
})
```
注意,如果在`watch`中直接调用`increment`方法,`this`指向的是`watcher`对象,而不是组件实例。因此,需要使用箭头函数来确保`this`指向组件实例。
vue父组件用watch方法监听子组件,关闭子组件页面,不使用$emit 方法,请写出相关代码
假设子组件的名称为`childComponent`,在父组件中监听子组件的变化,可以使用`$refs`来获取子组件的实例,然后通过`watch`方法监听子组件的变化。
以下是示例代码:
```
<template>
<div>
<button @click="showChildComponent = !showChildComponent">显示/隐藏子组件</button>
<child-component ref="childComponent" v-if="showChildComponent"></child-component>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
data() {
return {
showChildComponent: false
};
},
watch: {
showChildComponent: function(newValue) {
if (!newValue) {
// 关闭子组件页面的操作
this.$refs.childComponent.close();
}
}
}
};
</script>
```
在上述代码中,我们在父组件中定义了一个`showChildComponent`的布尔类型的数据,在点击按钮时改变它的值,从而控制子组件的显示和隐藏。通过`$refs`获取子组件的实例,然后在`watch`方法中监听`showChildComponent`的变化,当其为`false`时,调用子组件的`close()`方法,实现关闭子组件页面的操作。需要注意的是,`close()`方法需要在子组件中自行实现。
阅读全文
相关推荐
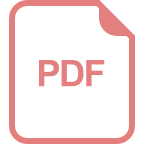
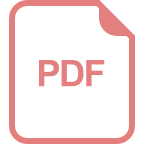
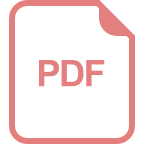
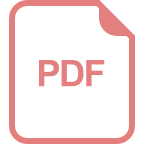
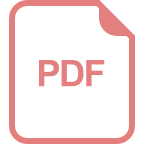







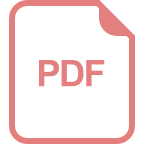
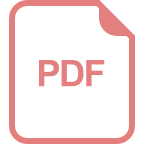


