geoserver通过rest自动发布postigs图层java示例
时间: 2023-08-22 10:08:20 浏览: 51
以下是使用 Java 代码通过 GeoServer REST API 自动发布 PostGIS 图层的示例:
```java
import java.io.IOException;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.Base64;
public class GeoServerPublisher {
private static final String GEOSERVER_URL = "http://localhost:8080/geoserver";
private static final String REST_URL = GEOSERVER_URL + "/rest";
private static final String WORKSPACE = "myworkspace";
private static final String DATASTORE = "mydatastore";
private static final String USERNAME = "admin";
private static final String PASSWORD = "geoserver";
public static void main(String[] args) throws IOException {
// Encode username and password as Base64 for authentication
String auth = USERNAME + ":" + PASSWORD;
String authHeader = "Basic " + Base64.getEncoder().encodeToString(auth.getBytes());
// Create workspace
createWorkspace("myworkspace", authHeader);
// Create datastore
createDatastore("mydatastore", authHeader);
// Publish PostGIS layer
publishPostgisLayer("myworkspace", "mydatastore", "mytable", "mylayer", authHeader);
}
private static void createWorkspace(String workspace, String authHeader) throws IOException {
URL url = new URL(REST_URL + "/workspaces");
HttpURLConnection con = (HttpURLConnection) url.openConnection();
con.setRequestMethod("POST");
con.setRequestProperty("Authorization", authHeader);
con.setRequestProperty("Content-Type", "application/xml");
con.setDoOutput(true);
String xml = "<workspace><name>" + workspace + "</name></workspace>";
con.getOutputStream().write(xml.getBytes());
int responseCode = con.getResponseCode();
if (responseCode != 201) {
throw new IOException("Failed to create workspace: " + con.getResponseMessage());
}
}
private static void createDatastore(String datastore, String authHeader) throws IOException {
URL url = new URL(REST_URL + "/workspaces/" + WORKSPACE + "/datastores");
HttpURLConnection con = (HttpURLConnection) url.openConnection();
con.setRequestMethod("POST");
con.setRequestProperty("Authorization", authHeader);
con.setRequestProperty("Content-Type", "application/xml");
con.setDoOutput(true);
String xml = "<dataStore><name>" + datastore + "</name><connectionParameters><host>localhost</host><port>5432</port><database>mydb</database><user>myuser</user><passwd>mypassword</passwd><dbtype>postgis</dbtype></connectionParameters></dataStore>";
con.getOutputStream().write(xml.getBytes());
int responseCode = con.getResponseCode();
if (responseCode != 201) {
throw new IOException("Failed to create datastore: " + con.getResponseMessage());
}
}
private static void publishPostgisLayer(String workspace, String datastore, String table, String layer, String authHeader) throws IOException {
URL url = new URL(REST_URL + "/workspaces/" + workspace + "/datastores/" + datastore + "/featuretypes");
HttpURLConnection con = (HttpURLConnection) url.openConnection();
con.setRequestMethod("POST");
con.setRequestProperty("Authorization", authHeader);
con.setRequestProperty("Content-Type", "application/xml");
con.setDoOutput(true);
String xml = "<featureType><name>" + layer + "</name><nativeName>" + table + "</nativeName></featureType>";
con.getOutputStream().write(xml.getBytes());
int responseCode = con.getResponseCode();
if (responseCode != 201) {
throw new IOException("Failed to publish layer: " + con.getResponseMessage());
}
}
}
```
请注意,这只是一个示例,并且需要根据您的实际情况进行修改。您需要将以下变量替换为您自己的值:
- GEOSERVER_URL: GeoServer 的 URL 地址。
- WORKSPACE: 工作空间名称。
- DATASTORE: 数据存储名称。
- USERNAME: GeoServer 管理员用户名。
- PASSWORD: GeoServer 管理员密码。
- createDatastore 方法中的连接参数:数据库主机名、端口、数据库名称、用户名和密码。
- publishPostgisLayer 方法中的表和图层名称。
还需要注意的是,此代码需要在 GeoServer 安装在本地计算机上且正在运行的情况下才能正常工作。如果 GeoServer 安装在远程服务器上,则需要更改 GEOSERVER_URL 变量为远程服务器的 URL 地址。
相关推荐
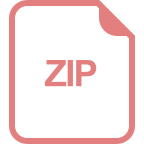













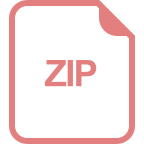