geoserver通过rest自动发布postigs图层的java示例,类型是application/json的
时间: 2023-11-27 15:51:29 浏览: 182
这里是一个使用Java代码发布PostGIS图层到GeoServer的示例:
```java
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class GeoServerRest {
public static void main(String[] args) throws IOException {
String geoServerUrl = "http://localhost:8080/geoserver";
String workspace = "workspace_name";
String storeName = "store_name";
String layerName = "layer_name";
String postgisHost = "localhost";
String postgisPort = "5432";
String postgisDatabase = "database_name";
String postgisSchema = "public";
String postgisUser = "username";
String postgisPass = "password";
// Create JSON payload for the new data store
String dataStorePayload = "{\n" +
" \"dataStore\": {\n" +
" \"name\": \"" + storeName + "\",\n" +
" \"connectionParameters\": {\n" +
" \"host\": \"" + postgisHost + "\",\n" +
" \"port\": \"" + postgisPort + "\",\n" +
" \"database\": \"" + postgisDatabase + "\",\n" +
" \"schema\": \"" + postgisSchema + "\",\n" +
" \"user\": \"" + postgisUser + "\",\n" +
" \"passwd\": \"" + postgisPass + "\",\n" +
" \"dbtype\": \"postgis\",\n" +
" \"max connections\": \"10\",\n" +
" \"min connections\": \"1\",\n" +
" \"fetch size\": \"1000\",\n" +
" \"Expose primary keys\": \"true\",\n" +
" \"Estimated extends\": \"true\",\n" +
" \"Connection timeout\": \"20\",\n" +
" \"validate connections\": \"true\",\n" +
" \"validate connections interval\": \"300\",\n" +
" \"preparedStatements\": \"false\",\n" +
" \"Support on the fly geometry simplification\": \"false\",\n" +
" \"JDBC virtual table name\": \"\",\n" +
" \"Loose bbox\": \"true\",\n" +
" \"Estimated extends factor\": \"0.1\",\n" +
" \"Fetch size\": \"1000\",\n" +
" \"Max open prepared statements\": \"50\",\n" +
" \"Min time between eviction runs millis\": \"5000\",\n" +
" \"Num tests per eviction run\": \"3\",\n" +
" \"Remove abandoned\": \"true\",\n" +
" \"Remove abandoned timeout\": \"300\"\n" +
" }\n" +
" }\n" +
"}";
// Create JSON payload for the new layer
String layerPayload = "{\n" +
" \"featureType\": {\n" +
" \"name\": \"" + layerName + "\",\n" +
" \"nativeName\": \"" + layerName + "\",\n" +
" \"title\": \"" + layerName + "\",\n" +
" \"srs\": \"EPSG:4326\",\n" +
" \"nativeCRS\": \"EPSG:4326\",\n" +
" \"enabled\": true,\n" +
" \"advertised\": true,\n" +
" \"metadata\": {\n" +
" \"entry\": [\n" +
" {\n" +
" \"@key\": \"cachingEnabled\",\n" +
" \"$\": false\n" +
" },\n" +
" {\n" +
" \"@key\": \"jai.enabled\",\n" +
" \"$\": true\n" +
" },\n" +
" {\n" +
" \"@key\": \"time\",\n" +
" \"$\": \"2015-05-14T10:36:11.672Z\"\n" +
" }\n" +
" ]\n" +
" },\n" +
" \"store\": {\n" +
" \"@class\": \"dataStore\",\n" +
" \"name\": \"" + storeName + "\",\n" +
" \"href\": \"" + geoServerUrl + "/rest/workspaces/" + workspace + "/datastores/" + storeName + ".json\"\n" +
" },\n" +
" \"maxFeatures\": 0,\n" +
" \"numDecimals\": 0,\n" +
" \"overridingServiceSRS\": false,\n" +
" \"skipNumberMatched\": false,\n" +
" \"circularArcPresent\": false,\n" +
" \"attributes\": {\n" +
" \"attribute\": [\n" +
" {\n" +
" \"name\": \"id\",\n" +
" \"minOccurs\": 0,\n" +
" \"maxOccurs\": 1,\n" +
" \"nillable\": true,\n" +
" \"binding\": \"java.lang.Integer\",\n" +
" \"length\": 10,\n" +
" \"precision\": null,\n" +
" \"defaultValue\": {\n" +
" \"$\": \"nextval('" + layerName + "_id_seq'::regclass)\",\n" +
" \"@type\": \"xsd:string\"\n" +
" }\n" +
" }\n" +
" ]\n" +
" },\n" +
" \"nativeBoundingBox\": {\n" +
" \"minx\": -180.0,\n" +
" \"maxx\": 180.0,\n" +
" \"miny\": -90.0,\n" +
" \"maxy\": 90.0,\n" +
" \"crs\": \"EPSG:4326\"\n" +
" },\n" +
" \"latLonBoundingBox\": {\n" +
" \"minx\": -180.0,\n" +
" \"maxx\": 180.0,\n" +
" \"miny\": -90.0,\n" +
" \"maxy\": 90.0,\n" +
" \"crs\": \"EPSG:4326\"\n" +
" },\n" +
" \"projectionPolicy\": \"FORCE_DECLARED\",\n" +
" \"enabledWMS\": false,\n" +
" \"circularArcPresent\": false,\n" +
" \"forcedDecimal\": false,\n" +
" \"styleName\": \"generic\"\n" +
" }\n" +
"}";
// Publish the new data store
URL dataStoreUrl = new URL(geoServerUrl + "/rest/workspaces/" + workspace + "/datastores.json");
HttpURLConnection dataStoreConn = (HttpURLConnection) dataStoreUrl.openConnection();
dataStoreConn.setRequestMethod("POST");
dataStoreConn.setDoOutput(true);
dataStoreConn.setRequestProperty("Content-Type", "application/json");
OutputStream dataStoreOutStream = dataStoreConn.getOutputStream();
dataStoreOutStream.write(dataStorePayload.getBytes());
dataStoreOutStream.flush();
// Get the response from the data store publish request
BufferedReader dataStoreReader = new BufferedReader(new InputStreamReader(dataStoreConn.getInputStream()));
String dataStoreResponse;
while ((dataStoreResponse = dataStoreReader.readLine()) != null) {
System.out.println(dataStoreResponse);
}
dataStoreReader.close();
dataStoreOutStream.close();
dataStoreConn.disconnect();
// Publish the new layer
URL layerUrl = new URL(geoServerUrl + "/rest/workspaces/" + workspace + "/datastores/" + storeName + "/featuretypes.json");
HttpURLConnection layerConn = (HttpURLConnection) layerUrl.openConnection();
layerConn.setRequestMethod("POST");
layerConn.setDoOutput(true);
layerConn.setRequestProperty("Content-Type", "application/json");
OutputStream layerOutStream = layerConn.getOutputStream();
layerOutStream.write(layerPayload.getBytes());
layerOutStream.flush();
// Get the response from the layer publish request
BufferedReader layerReader = new BufferedReader(new InputStreamReader(layerConn.getInputStream()));
String layerResponse;
while ((layerResponse = layerReader.readLine()) != null) {
System.out.println(layerResponse);
}
layerReader.close();
layerOutStream.close();
layerConn.disconnect();
}
}
```
请注意,此示例假定您已经在GeoServer中设置了PostGIS数据存储,并且您已经将所需的PostGIS驱动程序文件放置在GeoServer的类路径中。
阅读全文
相关推荐
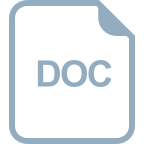
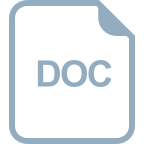
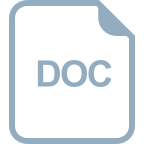

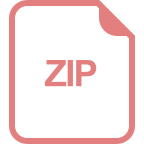
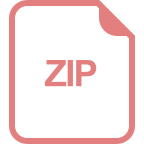
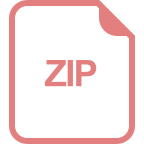
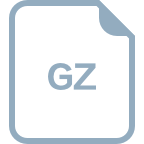










