import java.util.ArrayList;import java.util.Scanner;class Clothes { private String name; private String color; private int size; public Clothes(String name, String color, int size) { this.name = name; this.color = color; this.size = size; } public String getName() { return name; } public String getColor() { return color; } public int getSize() { return size; }}class Wardrobe { private ArrayList<Clothes> clothesList = new ArrayList<>(); public void addClothes(Clothes clothes) { clothesList.add(clothes); } public void removeClothes(int index) { clothesList.remove(index); } public void listClothes() { for (int i = 0; i < clothesList.size(); i++) { Clothes clothes = clothesList.get(i); System.out.println((i + 1) + ". " + clothes.getName() + " (" + clothes.getColor() + ", " + clothes.getSize() + ")"); } }}public class WardrobeManagementSystem { public static void main(String[] args) { Wardrobe wardrobe = new Wardrobe(); Scanner scanner = new Scanner(System.in); while (true) { System.out.println("请选择操作:"); System.out.println("1. 添加衣物"); System.out.println("2. 删除衣物"); System.out.println("3. 查看衣柜中的衣物"); System.out.println("4. 退出"); int choice = scanner.nextInt(); scanner.nextLine(); switch (choice) { case 1: System.out.println("请输入衣物名称:"); String name = scanner.nextLine(); System.out.println("请输入衣物颜色:"); String color = scanner.nextLine(); System.out.println("请输入衣物尺寸:"); int size = scanner.nextInt(); scanner.nextLine(); Clothes clothes = new Clothes(name, color, size); wardrobe.addClothes(clothes); System.out.println("衣物添加成功!"); break; case 2: System.out.println("请输入要删除的衣物编号:"); int index = scanner.nextInt() - 1; scanner.nextLine(); wardrobe.removeClothes(index); System.out.println("衣物删除成功!"); break; case 3: wardrobe.listClothes(); break; case 4: System.out.println("程序已退出!"); System.exit(0); default: System.out.println("输入有误,请重新输入!"); break; } } }}进行异常处理
时间: 2024-01-22 10:02:44 浏览: 29
在该程序中,可以使用 try-catch 语句对可能出现异常的部分进行处理。以下是可能出现异常的部分:
1. 在选择操作时,如果输入的不是数字,则会出现 InputMismatchException 异常。
2. 在删除衣物时,如果输入的编号超出了衣柜中衣物的数量,则会出现 IndexOutOfBoundsException 异常。
可以在 switch 语句外面添加一个 try 块来处理异常,如下所示:
```java
while (true) {
try {
System.out.println("请选择操作:");
System.out.println("1. 添加衣物");
System.out.println("2. 删除衣物");
System.out.println("3. 查看衣柜中的衣物");
System.out.println("4. 退出");
int choice = scanner.nextInt();
scanner.nextLine();
switch (choice) {
case 1:
System.out.println("请输入衣物名称:");
String name = scanner.nextLine();
System.out.println("请输入衣物颜色:");
String color = scanner.nextLine();
System.out.println("请输入衣物尺寸:");
int size = scanner.nextInt();
scanner.nextLine();
Clothes clothes = new Clothes(name, color, size);
wardrobe.addClothes(clothes);
System.out.println("衣物添加成功!");
break;
case 2:
System.out.println("请输入要删除的衣物编号:");
int index = scanner.nextInt() - 1;
scanner.nextLine();
wardrobe.removeClothes(index);
System.out.println("衣物删除成功!");
break;
case 3:
wardrobe.listClothes();
break;
case 4:
System.out.println("程序已退出!");
System.exit(0);
default:
System.out.println("输入有误,请重新输入!");
break;
}
} catch (InputMismatchException e) {
System.out.println("输入有误,请重新输入!");
scanner.nextLine();
} catch (IndexOutOfBoundsException e) {
System.out.println("输入的衣物编号不存在,请重新输入!");
scanner.nextLine();
}
}
```
在上面的代码中,使用了两个 catch 块来分别处理 InputMismatchException 和 IndexOutOfBoundsException 异常。如果出现了这些异常,程序会输出相应的错误信息,并要求用户重新输入。
相关推荐
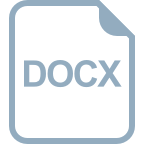
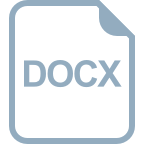
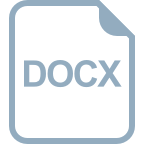
















