从文件in.txt读取信息,文件里的单词中间由空格隔开 统计每个单词出现的次数,从大到小排序后输出到文件out.txt 【输入形式】 文件里存储了多个单词,有重复,空格隔开 【输出形式】 从大到小输出单词及其出现的次数,次数一样的单词按字符串比较从小到大排序 【输入样例】in.txt文件里面的数据如下所示: hello world python hello word file number file file 【输出样例】out.txt文件里面的数据如下所示: file 3 hello 2 number 1 python 1 word 1 world 1
时间: 2024-02-19 14:03:54 浏览: 34
好的,我会帮你解决这个问题。
以下是 Python 代码实现:
```python
# 读取文件
with open('in.txt', 'r') as f:
words = f.read().split()
# 统计单词出现次数
word_dict = {}
for word in words:
if word in word_dict:
word_dict[word] += 1
else:
word_dict[word] = 1
# 按出现次数从大到小排序,次数一样的单词按字符串比较从小到大排序
sorted_words = sorted(word_dict.items(), key=lambda x: (-x[1], x[0]))
# 写入文件
with open('out.txt', 'w') as f:
for word, count in sorted_words:
f.write(f'{word} {count}\n')
```
你只需要将这段代码保存为 `word_count.py`,并将 `in.txt` 文件放在同一目录下,运行 `python word_count.py` 即可得到结果。
相关问题
从文件in.txt读取信息,文件里的单词中间由空格隔开 统计每个单词出现的次数,从大到小排序后输出到文件out.txt
好的,这是一个比较简单的文件操作和数据处理的问题。我可以提供一个简单的 Python 代码示例来实现这个功能。请将以下代码复制到一个名为 `word_count.py` 的文件中,并将 `in.txt` 文件放在同一目录下。
```python
# 打开文件并读取内容
with open('in.txt', 'r') as f:
content = f.read()
# 将文本分割成单词列表
words = content.split()
# 统计每个单词的出现次数
word_counts = {}
for word in words:
if word in word_counts:
word_counts[word] += 1
else:
word_counts[word] = 1
# 按照单词出现次数从大到小排序
sorted_word_counts = sorted(word_counts.items(), key=lambda x: x[1], reverse=True)
# 将结果写入文件
with open('out.txt', 'w') as f:
for word, count in sorted_word_counts:
f.write(f"{word}: {count}\n")
```
上面的代码首先打开文件 `in.txt` 并读取其中的内容,然后将内容分割成单词列表。接下来,它使用一个字典来统计每个单词的出现次数,并将结果按照单词出现次数从大到小排序。最后,它将结果写入文件 `out.txt`。运行代码后,你应该可以在当前目录下看到生成的 `out.txt` 文件,其中包含每个单词及其出现次数。
从文件in.txt读取信息,文件里的单词中间由空格隔开,统计每个单词出现的次数,从大到小排序后输出到文件out.txt
好的,我可以帮你写一个Python程序来完成这个任务。请确保当前目录下有in.txt文件,并且out.txt文件不存在。以下是程序代码:
```python
# 读取文件内容
with open("in.txt", "r") as f:
content = f.read()
# 统计单词出现次数
word_count = {}
for word in content.split():
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
# 按照出现次数排序
sorted_word_count = sorted(word_count.items(), key=lambda x: x[1], reverse=True)
# 将结果输出到文件
with open("out.txt", "w") as f:
for word, count in sorted_word_count:
f.write(f"{word} {count}\n")
```
程序首先读取in.txt文件的内容,然后统计每个单词出现的次数,存储在一个字典中。接着,使用Python内置的sorted函数按照出现次数对字典进行排序,并将结果输出到out.txt文件中。
注意,如果in.txt文件中有多个空格或者换行符,程序可能会将它们当作一个单词的一部分。如果需要更精确的处理,可以使用正则表达式或者第三方库如NLTK来进行分词。
相关推荐
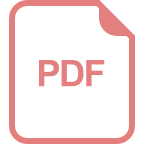
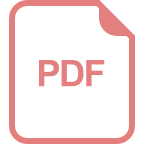










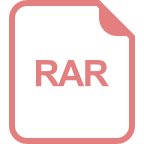
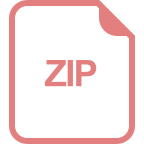
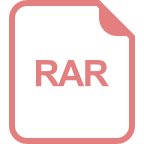
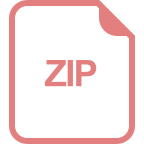