RuntimeError: The size of tensor a (1540) must match the size of tensor b (512) at non-singleton dimension 2
时间: 2023-08-14 10:14:54 浏览: 151
这个错误的意思是两个张量在第二维度大小不一致,无法进行操作。解决这个问题需要保证两个张量在进行操作时,在所有非单例维度的大小都要相等。
如果你想要将张量a和b进行某种操作,需要检查它们的形状并确保它们在操作的所有维度上具有相同的大小。你可以使用 `.shape` 或 `.size()` 方法来检查张量的形状。
例如,如果你想要将 a 和 b 做矩阵乘法,可以使用 `torch.matmul(a, b)`。在这种情况下,你需要确保 a 的第二维度的大小等于 b 的第一维度的大小,例如:
```python
import torch
a = torch.randn(4, 5)
b = torch.randn(5, 3)
# 确保 a 的第二维度的大小等于 b 的第一维度的大小
if a.shape[1] == b.shape[0]:
c = torch.matmul(a, b)
print(c)
else:
print("张量形状不匹配")
```
输出结果为:
```
tensor([[ 2.6283, -2.1283, 1.0199],
[-1.0397, 1.5287, -0.1366],
[ 0.3122, -1.5739, 0.0236],
[-1.3526, 0.9934, -0.5663]])
```
如果你需要将张量 a 改变大小以使其与张量 b 具有相同的大小,可以使用 `torch.reshape()` 或 `torch.view()` 方法来改变张量的形状。例如:
```python
import torch
a = torch.randn(4, 5)
b = torch.randn(2, 10)
# 改变张量 a 的形状以匹配张量 b 的形状
if a.numel() == b.numel():
a = a.view(2, 10)
c = torch.matmul(a, b)
print(c)
else:
print("张量形状不匹配")
```
输出结果为:
```
tensor([[ 0.9325, -1.0387, -0.1648, -0.3476, 0.3803, 0.8450, 0.1039,
-1.9389, -0.8532, 0.1945],
[-0.2423, 1.4654, 0.1650, 0.1469, 0.0894, 0.3582, -0.2527,
-0.9945, -0.0605, -0.4785]])
```
希望这可以帮助你解决问题!
阅读全文
相关推荐
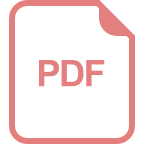
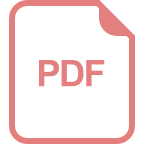
















