根据上述语法规则构造递归下降分析程序
时间: 2023-08-21 22:24:23 浏览: 85
好的,我可以帮你构造递归下降分析程序。首先,根据语法规则,可以将其转换为如下的语法规则:
```
S -> A+B
A -> a*A | a
B -> b*B | b
```
其中,S、A、B 都是非终结符,a、b 是终结符。
接下来,我们可以按照递归下降分析的方法,分别对每个非终结符编写一个函数。具体实现如下:
```python
# 定义全局变量
pos = 0 # 记录当前处理到的位置
text = "" # 记录要分析的文本
def match(expected):
"""匹配终结符"""
global pos
if pos < len(text) and text[pos] == expected:
pos += 1
else:
raise Exception("Syntax Error: expected {} but got {}".format(expected, text[pos]))
def A():
"""处理 A"""
global pos
if pos < len(text) and text[pos] == "a":
match("a")
if pos < len(text) and text[pos] == "*":
match("*")
A()
else:
pass
else:
raise Exception("Syntax Error: expected a but got {}".format(text[pos]))
def B():
"""处理 B"""
global pos
if pos < len(text) and text[pos] == "b":
match("b")
if pos < len(text) and text[pos] == "B":
B()
else:
pass
else:
raise Exception("Syntax Error: expected b but got {}".format(text[pos]))
def S():
"""处理 S"""
A()
match("+")
B()
def parse(input_text):
"""解析输入文本"""
global pos, text
pos = 0
text = input_text
S()
if pos == len(text):
print("Parsing Succeeded")
else:
raise Exception("Syntax Error: unexpected end of input")
```
在上述代码中,我们定义了全局变量 `pos` 和 `text`,分别表示当前处理到的位置和要分析的文本。然后,我们编写了三个函数 `A()`、`B()` 和 `S()`,分别对应非终结符 A、B 和 S。
在 `A()` 和 `B()` 函数中,我们使用了递归的方法,不断调用自身,处理重复出现的子表达式。
最后,我们编写了一个 `parse()` 函数,用来解析输入文本。在解析过程中,我们首先调用 `S()` 函数,然后检查是否已经处理完了整个输入文本。
使用时,只需要调用 `parse()` 函数,传入要分析的文本即可。例如,对于输入文本 `"a*a+aabb"`,我们可以这样调用:
```python
parse("a*a+aabb")
```
程序将会输出 `"Parsing Succeeded"`,表示分析成功。如果输入的文本不符合语法规则,则会抛出异常,给出相应的错误信息。
阅读全文
相关推荐
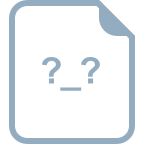
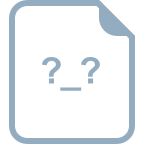


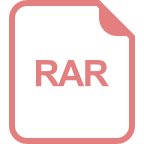
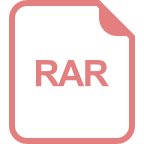
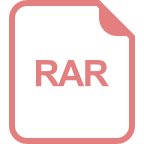
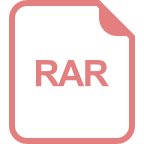
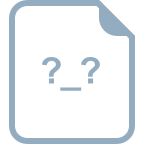
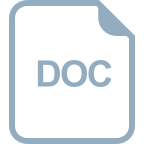
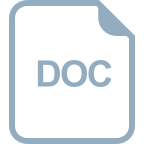
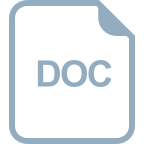
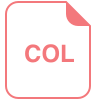
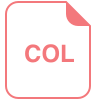
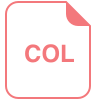
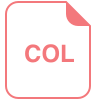


