用Python实现陷波滤波器复原图像的代码
时间: 2024-04-07 13:07:36 浏览: 288
陷波滤波器可以用于去除图像中的周期性噪声,其原理是在频域中将指定频率范围内的信号滤除。
以下是使用Python实现陷波滤波器复原图像的代码:
```python
import cv2
import numpy as np
import matplotlib.pyplot as plt
# 加载图像
img = cv2.imread('image.jpg', cv2.IMREAD_GRAYSCALE)
# 计算图像的傅里叶变换
f = np.fft.fft2(img)
fshift = np.fft.fftshift(f)
# 构造陷波滤波器
rows, cols = img.shape
crow, ccol = rows/2, cols/2
mask = np.ones((rows, cols), np.uint8)
r = 30
center = [crow, ccol]
x, y = np.ogrid[:rows, :cols]
mask_area = (x - center[0])**2 + (y - center[1])**2 <= r**2
mask[mask_area] = 0
# 应用陷波滤波器
fshift = fshift * mask
f_ishift = np.fft.ifftshift(fshift)
img_back = np.fft.ifft2(f_ishift)
img_back = np.abs(img_back)
# 显示结果
plt.subplot(121), plt.imshow(img, cmap='gray')
plt.title('Input Image'), plt.xticks([]), plt.yticks([])
plt.subplot(122), plt.imshow(img_back, cmap='gray')
plt.title('Result'), plt.xticks([]), plt.yticks([])
plt.show()
```
在上述代码中,我们首先加载图像,并计算图像的傅里叶变换。然后构造一个陷波滤波器,该滤波器将指定半径范围内的信号滤除。最后,我们将该滤波器应用于图像的傅里叶变换中,并对结果进行傅里叶逆变换以得到复原图像。
阅读全文
相关推荐
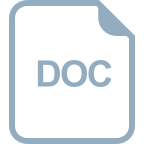
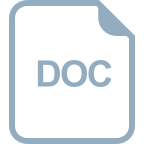
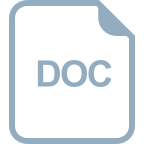


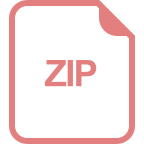
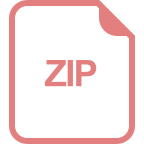
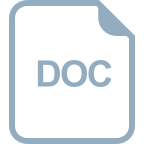


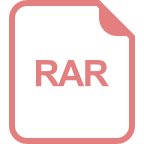





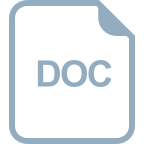
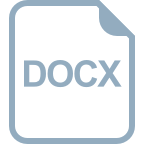