C语言,输入N个学生c语言成绩,请你设计一个函数编程找最高分,并列第一学生数
时间: 2024-01-25 16:04:42 浏览: 33
好的,以下是用 C 语言实现找最高分和最高分学生数的函数:
```c
#include <stdio.h>
void find_highest_score(int scores[], int num_of_students, int *highest_score, int *num_of_best_students)
{
int i;
*highest_score = scores[0];
*num_of_best_students = 1;
for (i = 1; i < num_of_students; i++) {
if (scores[i] > *highest_score) {
*highest_score = scores[i];
*num_of_best_students = 1;
} else if (scores[i] == *highest_score) {
(*num_of_best_students)++;
}
}
}
```
这个函数的参数包括一个整型数组 `scores`,存储了学生的成绩;一个整数 `num_of_students`,表示学生人数;以及两个指向整数的指针 `highest_score` 和 `num_of_best_students`,用于返回最高分和最高分学生数。
函数的实现使用了一个循环,遍历整个成绩数组,同时通过指针更新最高分和最高分学生数。具体来说,我们先将 `highest_score` 初始化为数组的第一个元素,将 `num_of_best_students` 初始化为 1(因为第一个学生是当前最高分的学生);然后从数组的第二个元素开始遍历,如果当前学生的成绩比当前最高分高,就更新 `highest_score` 和 `num_of_best_students`;如果当前学生的成绩等于当前最高分,就将 `num_of_best_students` 加 1。
调用这个函数的方法如下:
```c
int main()
{
int scores[] = {86, 92, 77, 92, 95, 86, 91};
int num_of_students = 7;
int highest_score, num_of_best_students;
find_highest_score(scores, num_of_students, &highest_score, &num_of_best_students);
printf("The highest score is %d\n", highest_score);
printf("The number of best students is %d\n", num_of_best_students);
return 0;
}
```
这个示例程序中,我们先定义了一个成绩数组 `scores`,然后调用 `find_highest_score()` 函数计算最高分和最高分学生数,并使用 `printf()` 函数输出结果。
相关推荐
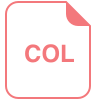
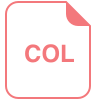
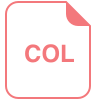
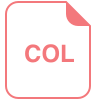










