用C语言写一个通用性强的链表
时间: 2023-12-29 20:21:24 浏览: 24
以下是用C语言实现的一个通用性强的链表:
```c
#include <stdio.h>
#include <stdlib.h>
struct node {
void *data;
struct node *next;
};
typedef struct node node_t;
node_t *create_node(void *data) {
node_t *node = (node_t*)malloc(sizeof(node_t));
node->data = data;
node->next = NULL;
return node;
}
void insert_node(node_t **head, node_t *node) {
if (*head == NULL) {
*head = node;
} else {
node_t *current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = node;
}
}
void delete_node(node_t **head, node_t *node) {
if (*head == node) {
*head = node->next;
} else {
node_t *current = *head;
while (current != NULL && current->next != node) {
current = current->next;
}
if (current != NULL) {
current->next = node->next;
}
}
free(node);
}
void traverse(node_t *head, void (*callback)(void *)) {
node_t *current = head;
while (current != NULL) {
callback(current->data);
current = current->next;
}
}
void destroy_list(node_t **head) {
while (*head != NULL) {
node_t *current = *head;
*head = current->next;
free(current);
}
}
```
这个链表可以存储任意类型的数据,因为它使用了 void* 指针来存储数据。在使用时,只需要把需要存储的数据转换成 void* 类型即可。例如,如果要存储一个整数,可以这样做:
```c
int *data = (int*)malloc(sizeof(int));
*data = 10;
node_t *node = create_node(data);
```
这个链表支持插入、删除、遍历和销毁等基本操作。例如,要遍历整个链表并打印出每个节点的数据,可以这样做:
```c
void print_data(void *data) {
int *value = (int*)data;
printf("%d ", *value);
}
int main() {
node_t *head = NULL;
int *data1 = (int*)malloc(sizeof(int));
int *data2 = (int*)malloc(sizeof(int));
int *data3 = (int*)malloc(sizeof(int));
*data1 = 10;
*data2 = 20;
*data3 = 30;
insert_node(&head, create_node(data1));
insert_node(&head, create_node(data2));
insert_node(&head, create_node(data3));
traverse(head, print_data);
destroy_list(&head);
return 0;
}
```
输出结果为:
```
10 20 30
```
可以看到,这个链表非常通用,可以存储任何类型的数据,并支持常用的操作。
相关推荐
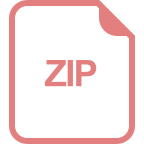
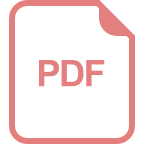
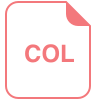
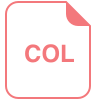
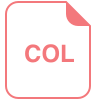
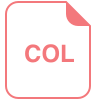
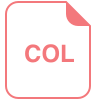









