import pandas as pd from sklearn.ensemble import RandomForestClassifier from sklearn.metrics import accuracy_score, confusion_matrix,classification_report import seaborn as sns import matplotlib.pyplot as plt # 读取数据 data = pd.read_excel('E:/桌面/预测脆弱性/20230523/预测样本/预测样本.xlsx') # 分割训练集和验证集 train_data = data.sample(frac=0.8, random_state=1) test_data = data.drop(train_data.index) # 定义特征变量和目标变量 features = ['高程', '起伏度', '桥梁长', '道路长', '平均坡度', '平均地温', 'T小于0', '相态'] target = '交通风险' # 训练随机森林模型 rf = RandomForestClassifier(n_estimators=100, random_state=1) rf.fit(train_data[features], train_data[target]) # 在验证集上进行预测并计算精度、召回率和F1值等指标 pred = rf.predict(test_data[features]) accuracy = accuracy_score(test_data[target], pred) confusion_mat = confusion_matrix(test_data[target], pred) classification_rep = classification_report(test_data[target], pred) print('Accuracy:', accuracy) print('Confusion matrix:') print(confusion_mat) print('Classification report:') print(classification_rep) # 输出混淆矩阵图片 sns.heatmap(confusion_mat, annot=True, cmap="Blues") plt.show() # 读取新数据文件并预测结果 new_data = pd.read_excel('E:/桌面/预测脆弱性/20230523/预测样本/预测结果/交通风险预测096.xlsx') new_pred = rf.predict(new_data[features]) new_data['交通风险预测结果'] = new_pred new_data.to_excel('E:/桌面/预测脆弱性/20230523/预测样本/预测结果/交通风险预测096结果.xlsx', index=False)修改代码使得显示决策树模型以及多分类的roc曲线和auc值
时间: 2024-02-18 19:00:48 浏览: 98
首先需要说明的一点是,随机森林模型是一个集成学习方法,其中包含了多个决策树模型。因此,如果要显示决策树模型,需要先在随机森林模型中选择一个决策树进行可视化。下面是修改后的代码,其中包括显示决策树模型和多分类的ROC曲线和AUC值:
```
import pandas as pd
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score, confusion_matrix, classification_report, roc_curve, auc
import seaborn as sns
import matplotlib.pyplot as plt
# 读取数据
data = pd.read_excel('E:/桌面/预测脆弱性/20230523/预测样本/预测样本.xlsx')
# 分割训练集和验证集
train_data = data.sample(frac=0.8, random_state=1)
test_data = data.drop(train_data.index)
# 定义特征变量和目标变量
features = ['高程', '起伏度', '桥梁长', '道路长', '平均坡度', '平均地温', 'T小于0', '相态']
target = '交通风险'
# 训练随机森林模型
rf = RandomForestClassifier(n_estimators=100, random_state=1)
rf.fit(train_data[features], train_data[target])
# 显示决策树模型
estimator = rf.estimators_[0]
plt.figure(figsize=(20,20))
tree.plot_tree(estimator, feature_names=features, filled=True)
plt.show()
# 在验证集上进行预测并计算精度、召回率和F1值等指标
pred = rf.predict(test_data[features])
accuracy = accuracy_score(test_data[target], pred)
confusion_mat = confusion_matrix(test_data[target], pred)
classification_rep = classification_report(test_data[target], pred)
print('Accuracy:', accuracy)
print('Confusion matrix:')
print(confusion_mat)
print('Classification report:')
print(classification_rep)
# 输出混淆矩阵图片
sns.heatmap(confusion_mat, annot=True, cmap="Blues")
plt.show()
# 计算多分类的ROC曲线和AUC值
n_classes = len(set(data[target]))
y_test = pd.get_dummies(test_data[target]).values
y_score = rf.predict_proba(test_data[features])
fpr = dict()
tpr = dict()
roc_auc = dict()
for i in range(n_classes):
fpr[i], tpr[i], _ = roc_curve(y_test[:, i], y_score[:, i])
roc_auc[i] = auc(fpr[i], tpr[i])
# 绘制ROC曲线
plt.figure()
colors = ['red', 'green', 'blue', 'orange']
for i, color in zip(range(n_classes), colors):
plt.plot(fpr[i], tpr[i], color=color, label='ROC curve of class {0} (AUC = {1:0.2f})'.format(i, roc_auc[i]))
plt.plot([0, 1], [0, 1], 'k--')
plt.xlim([0.0, 1.0])
plt.ylim([0.0, 1.05])
plt.xlabel('False Positive Rate')
plt.ylabel('True Positive Rate')
plt.title('Multi-class ROC curves')
plt.legend(loc="lower right")
plt.show()
# 读取新数据文件并预测结果
new_data = pd.read_excel('E:/桌面/预测脆弱性/20230523/预测样本/预测结果/交通风险预测096.xlsx')
new_pred = rf.predict(new_data[features])
new_data['交通风险预测结果'] = new_pred
new_data.to_excel('E:/桌面/预测脆弱性/20230523/预测样本/预测结果/交通风险预测096结果.xlsx', index=False)
```
修改后的代码中,首先使用`estimators_`属性选择了随机森林模型中的一个决策树,然后使用`plot_tree`函数对其进行可视化。接着,计算多分类的ROC曲线和AUC值,并使用`roc_curve`和`auc`函数分别计算每个类别的ROC曲线和AUC值。最后,使用`plt.plot`函数绘制多分类的ROC曲线,并在图例中显示每个类别的AUC值。
阅读全文
相关推荐
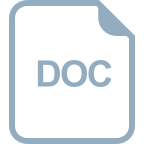
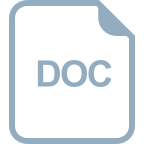
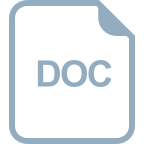



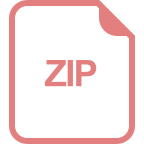
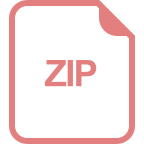
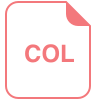
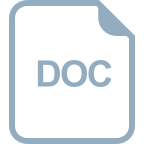
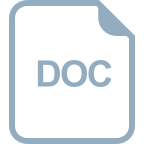
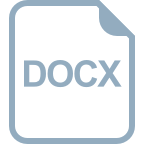
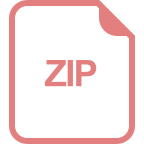