使用PHP +js +html 实现 网页上快速读取 并每五秒实时打印超大文件日志内容
时间: 2023-12-20 09:07:19 浏览: 160
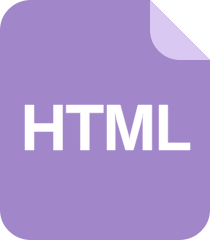
在网页端实现文件信息的自动读取
可以使用PHP的fread函数读取文件内容,然后通过JS实时将内容显示在网页上。具体实现步骤如下:
1. 创建一个HTML页面,包含一个文本框和一个按钮,用于输入文件路径和开始读取文件。
```
<!DOCTYPE html>
<html>
<head>
<title>快速读取超大文件日志</title>
<meta charset="utf-8">
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script>
function startReading() {
var filepath = document.getElementById("filepath").value;
$.ajax({
url: 'readfile.php',
type: 'POST',
data: {filepath: filepath},
success: function(response) {
$('#logcontent').html(response);
}
});
setInterval(function(){
$.ajax({
url: 'readfile.php',
type: 'POST',
data: {filepath: filepath, lastpos: $('#lastpos').val()},
success: function(response) {
$('#logcontent').append(response);
$('#lastpos').val($('#logcontent').text().length);
}
});
}, 5000);
}
</script>
</head>
<body>
<h1>快速读取超大文件日志</h1>
<label for="filepath">文件路径:</label>
<input type="text" id="filepath" name="filepath" size="50">
<button onclick="startReading()">开始读取</button>
<hr>
<div id="logcontent"></div>
<input type="hidden" id="lastpos" name="lastpos" value="0">
</body>
</html>
```
2. 创建一个PHP文件readfile.php,用于读取文件内容并返回给JS。为了实现每五秒自动刷新,可以在此文件中使用PHP的fseek函数定位到文件末尾,并通过读取上次结束位置和当前位置之间的内容来获取新增的日志内容。
```
<?php
if(isset($_POST['filepath'])) {
$filepath = $_POST['filepath'];
$lastpos = isset($_POST['lastpos']) ? $_POST['lastpos'] : 0;
$logcontent = '';
$filesize = filesize($filepath);
if($filesize > $lastpos) {
$file = fopen($filepath, 'r');
fseek($file, $lastpos);
while(!feof($file)) {
$logcontent .= fgets($file);
}
fclose($file);
}
echo nl2br($logcontent);
}
?>
```
3. 在PHP的配置文件php.ini中将output_buffering设置为Off,以便实现无缓冲输出。
```
output_buffering = Off
```
这样,当用户输入文件路径并点击“开始读取”按钮时,JS会向readfile.php发送POST请求,读取并显示文件内容,并且每五秒会自动刷新页面,读取并显示新增的日志内容。
阅读全文
相关推荐







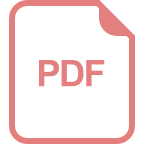
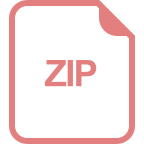
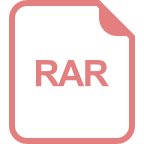
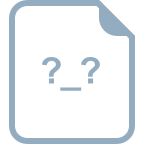
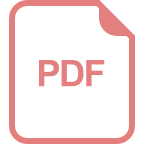
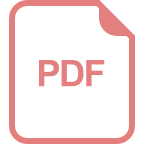
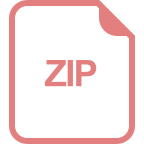
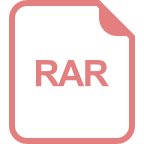
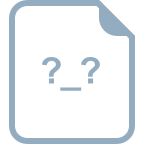