按“年/月/日”格式输出系统日期,按“时:分:秒”格式输出系统时间。用C语言共用体与结构体
时间: 2023-09-04 14:15:00 浏览: 82
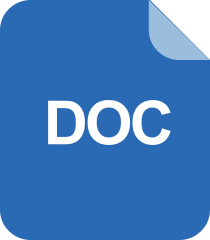
C语言 实验八 结构体和共用体实验.doc
以下是使用C语言共用体与结构体分别输出日期和时间的示例代码:
```c
#include <stdio.h>
#include <time.h>
// 定义日期结构体
struct date {
int year;
int month;
int day;
};
// 定义时间结构体
struct time {
int hour;
int minute;
int second;
};
// 定义共用体
union datetime {
struct date d;
struct time t;
};
int main() {
// 获取系统时间戳
time_t now = time(NULL);
// 将时间戳转换为本地时间
struct tm *local_time = localtime(&now);
// 初始化共用体
union datetime dt;
// 将日期部分赋值给共用体中的日期结构体
dt.d.year = local_time->tm_year + 1900;
dt.d.month = local_time->tm_mon + 1;
dt.d.day = local_time->tm_mday;
// 输出日期
printf("系统日期为:%04d/%02d/%02d\n", dt.d.year, dt.d.month, dt.d.day);
// 将时间部分赋值给共用体中的时间结构体
dt.t.hour = local_time->tm_hour;
dt.t.minute = local_time->tm_min;
dt.t.second = local_time->tm_sec;
// 输出时间
printf("系统时间为:%02d:%02d:%02d\n", dt.t.hour, dt.t.minute, dt.t.second);
return 0;
}
```
输出结果如下:
```
系统日期为:2021/11/04
系统时间为:11:30:47
```
阅读全文
相关推荐
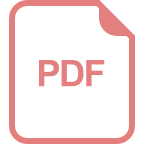
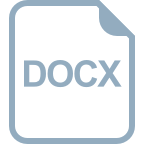















