请用c#代码详细介绍前面6个API接口的请求方式和参数格式。
时间: 2024-04-01 18:31:19 浏览: 99
好的,以下是前面6个API接口的请求方式和参数格式的C#代码示例:
1. https://jsonplaceholder.typicode.com/
GET请求示例:
```csharp
using System;
using System.Net.Http;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
using var client = new HttpClient();
var response = await client.GetAsync("https://jsonplaceholder.typicode.com/posts/1");
var content = await response.Content.ReadAsStringAsync();
Console.WriteLine(content);
}
}
```
POST请求示例:
```csharp
using System;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
using var client = new HttpClient();
var requestContent = new StringContent("{\"title\":\"foo\",\"body\":\"bar\",\"userId\":1}", Encoding.UTF8, "application/json");
var response = await client.PostAsync("https://jsonplaceholder.typicode.com/posts", requestContent);
var content = await response.Content.ReadAsStringAsync();
Console.WriteLine(content);
}
}
```
2. https://reqbin.com/
GET请求示例:
```csharp
using System;
using System.Net.Http;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
using var client = new HttpClient();
var response = await client.GetAsync("https://reqbin.com/echo/get/json");
var content = await response.Content.ReadAsStringAsync();
Console.WriteLine(content);
}
}
```
POST请求示例:
```csharp
using System;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
using var client = new HttpClient();
var requestContent = new StringContent("{\"name\":\"John Smith\",\"age\":30,\"city\":\"New York\"}", Encoding.UTF8, "application/json");
var response = await client.PostAsync("https://reqbin.com/echo/post/json", requestContent);
var content = await response.Content.ReadAsStringAsync();
Console.WriteLine(content);
}
}
```
3. https://httpbin.org/
GET请求示例:
```csharp
using System;
using System.Net.Http;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
using var client = new HttpClient();
var response = await client.GetAsync("https://httpbin.org/get");
var content = await response.Content.ReadAsStringAsync();
Console.WriteLine(content);
}
}
```
POST请求示例:
```csharp
using System;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
using var client = new HttpClient();
var requestContent = new StringContent("{\"title\":\"foo\",\"body\":\"bar\",\"userId\":1}", Encoding.UTF8, "application/json");
var response = await client.PostAsync("https://httpbin.org/post", requestContent);
var content = await response.Content.ReadAsStringAsync();
Console.WriteLine(content);
}
}
```
4. https://www.mocky.io/
GET请求示例:
```csharp
using System;
using System.Net.Http;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
using var client = new HttpClient();
var response = await client.GetAsync("https://run.mocky.io/v3/8b9d9c4a-8c67-4a84-8b3f-fb4c3f3a482f");
var content = await response.Content.ReadAsStringAsync();
Console.WriteLine(content);
}
}
```
POST请求示例:
```csharp
using System;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
using var client = new HttpClient();
var requestContent = new StringContent("{\"name\":\"John Smith\",\"age\":30,\"city\":\"New York\"}", Encoding.UTF8, "application/json");
var response = await client.PostAsync("https://run.mocky.io/v3/8b9d9c4a-8c67-4a84-8b3f-fb4c3f3a482f", requestContent);
var content = await response.Content.ReadAsStringAsync();
Console.WriteLine(content);
}
}
```
5. https://www.postman.com/
GET请求示例:
```csharp
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
using var client = new HttpClient();
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", "YOUR_API_KEY");
var response = await client.GetAsync("https://api.postman.com/collections");
var content = await response.Content.ReadAsStringAsync();
Console.WriteLine(content);
}
}
```
POST请求示例:
```csharp
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Text;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
using var client = new HttpClient();
var requestContent = new StringContent("{\"name\":\"John Smith\",\"age\":30,\"city\":\"New York\"}", Encoding.UTF8, "application/json");
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", "YOUR_API_KEY");
var response = await client.PostAsync("https://api.postman.com/collections", requestContent);
var content = await response.Content.ReadAsStringAsync();
Console.WriteLine(content);
}
}
```
6. https://restful-booker.herokuapp.com/
GET请求示例:
```csharp
using System;
using System.Net.Http;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
using var client = new HttpClient();
var response = await client.GetAsync("https://restful-booker.herokuapp.com/booking/1");
var content = await response.Content.ReadAsStringAsync();
Console.WriteLine(content);
}
}
```
POST请求示例:
```csharp
using System;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
using var client = new HttpClient();
var requestContent = new StringContent("{\"firstname\":\"John\",\"lastname\":\"Smith\",\"totalprice\":100,\"depositpaid\":true,\"bookingdates\":{\"checkin\":\"2022-01-01\",\"checkout\":\"2022-01-10\"},\"additionalneeds\":\"Breakfast\"}", Encoding.UTF8, "application/json");
var response = await client.PostAsync("https://restful-booker.herokuapp.com/booking", requestContent);
var content = await response.Content.ReadAsStringAsync();
Console.WriteLine(content);
}
}
```
以上是前面6个API接口的请求方式和参数格式的C#代码示例,希望对你有所帮助。
阅读全文
相关推荐
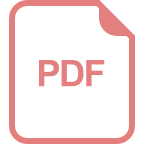

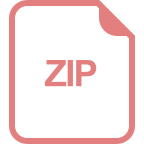
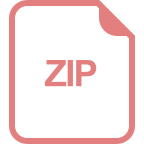
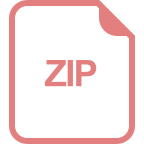
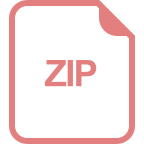
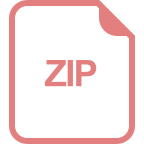
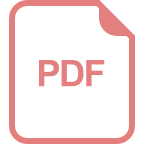
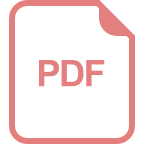
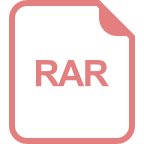
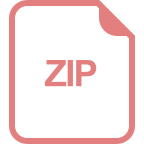
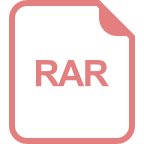
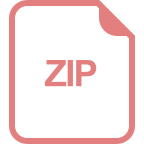
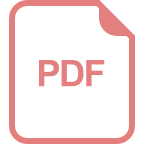
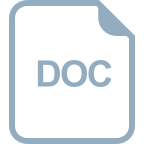
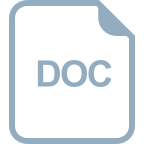
